Why You Should Always Use Option Explicit in VBA
Don't let typos and logic errors ruin your VBA code. Read our latest blog post to learn about the importance of Option Explicit and how to use it.
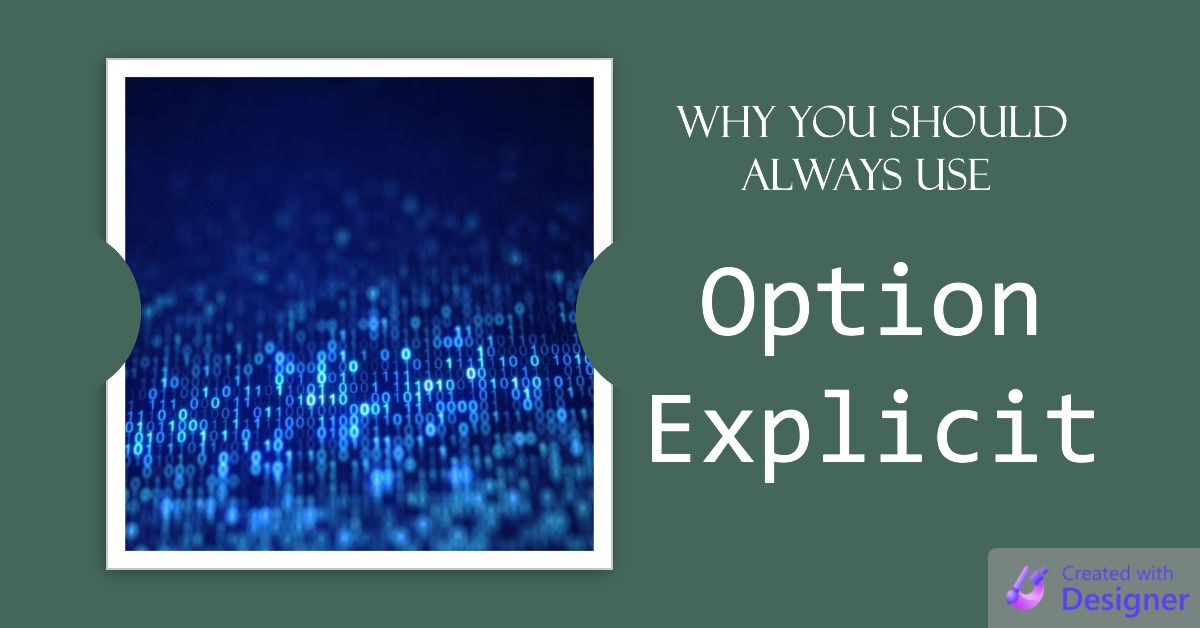
In an apparent attempt to be more "beginner friendly", VBA does not require you to define variables before you refer to them.
Instead, you can create variables implicitly as you assign values to them. The alternative is to explicitly declare variables via the Dim
keyword.
'-- Implicit variable creation --
For i = 1 To 3
Debug.Print i
Next i
i
is implicitly created in the line For i = 1 To 3
.'-- Explicit variable creation --
Dim i As Integer
For i = 1 to 3
Debug.Print i
Next i
i
is explicitly created in the line Dim i As Integer
.You should always explicitly declare your variables in VBA.
The Impact of Typos and Logic Errors
Typos are a common mistake that programmers make.
In VBA, typos can be particularly dangerous because they can lead to logic errors that are difficult to detect. For example, suppose you want to create a variable called "count" to keep track of how many times a loop has executed. If you accidentally type "conut" instead of "count," VBA will create a new variable called "conut" and use it in your code. However, since "conut" is not what you intended, your program will not work as expected.
Here is an example:
Sub Example()
Dim count As Integer
For i = 1 To 5
counit = count + i
MsgBox "The value of count is " & count
Next i
End Sub
In this example, we intend to create a variable called "count." However, we accidentally typed "counit" instead. VBA creates a new variable called "counit" and uses it in our code. As a result, the value of "count" never changes, and the program displays the same message five times.
Implicit Variable Creation in Other Languages
VBA is not the only programming language that allows implicit variable creation.
Many other languages, such as JavaScript, Python, and Ruby, also allow you to use variables without declaring them first. In other words, VBA is not way out on a limb with this design decision.
What is Option Explicit?
Option Explicit is a VBA statement that forces you to declare all variables before using them.
By default, VBA allows you to use variables without declaring them first (so-called "implicit declaration" as described above). While this might seem convenient, it can lead to errors and bugs in your code. When you use Option Explicit, VBA will generate an error message if you try to use an undeclared variable.
Option Explicit
Used at the module level to force explicit declaration of all variables in that module.
...
When Option Explicit appears in a module, you must explicitly declare all variables by using the Dim, Private, Public, ReDim, or Static statements. If you attempt to use an undeclared variable name, an error occurs at compile time.
Benefits of Option Explicit
Here are a few of the benefits of using Option Explicit
:
- It prevents typos and other spelling errors from creating new variables.
- It helps catch logic errors early in the development process.
- It makes your code easier to read and understand.
- It improves the reliability and maintainability of your code.
- It reduces the time and effort required to debug your code.
- It is considered best practice in VBA programming (which helps signal to other developers that you know what you are doing).
Turning on Option Explicit by Default
Now that we've seen some of the advantages of using Option Explicit, you're probably eager to start using it.
You could just type the words Option Explicit
in the header of every new standard module, class module, or form or report object in your database. That would be tedious. It would also be too easy to forget to include that line in a new module.
The better alternative is to enable the option to add Option Explicit
to every new module via Tools > Options > Editor > [√] Require Variable Declaration.
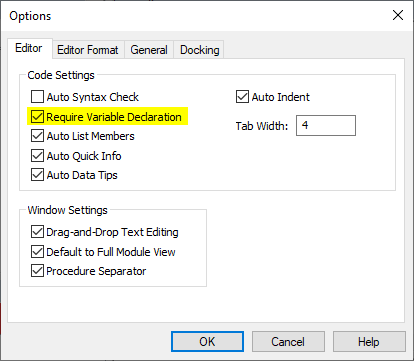
Option Explicit
in its header.IMPORTANT NOTE: This will only affect future code modules that you create. Existing modules will not be changed.
Conclusion
Option Explicit is a powerful feature of VBA that helps prevent typos and logic errors in your code.
By forcing you to declare all variables before using them, it improves the reliability and maintainability of your code. While other programming languages allow implicit variable creation, it is always a good idea to declare your variables explicitly to prevent bugs and errors.
To help with this, be sure to enable the setting Require variable declaration in all of your development environments.