The vbNullChar Constant in VBA
What is the vbNullChar constant in VBA and how does it differ from vbNullString?
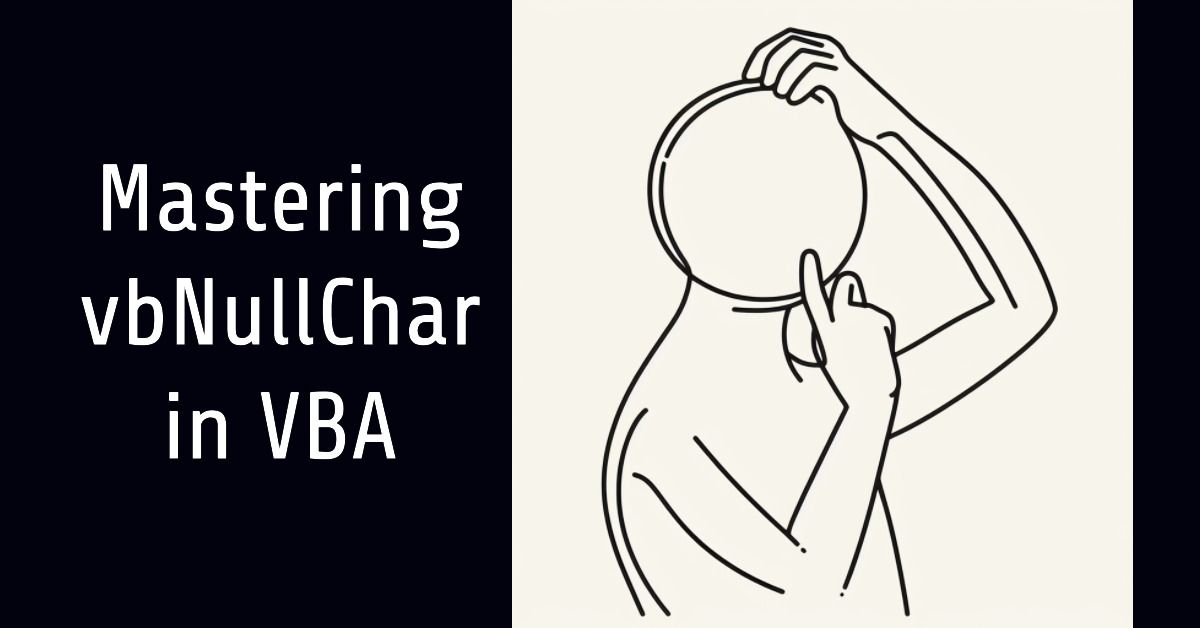
vbNullString is one of many ways to express the concept of nothingness in VBA. For the others, check out An Article About Nothing.
vbNullChar According to the VBA Spec
According to section 6.1.2.2–Constants Module–of the VBA Language Specification, the constant vbNullChar
is a constant representing the following value:
VBA.Strings.Chr$(0)
Just like the vbNullString
we've previously explored, vbNullChar
represents another aspect of the concept of nothingness in VBA. To better understand it and its use cases, let's dive deeper into this constant.
vbNullChar: What Is It?
vbNullChar
is a constant in VBA that represents a character with an ASCII code of zero.
You can also obtain this character using Chr(0)
. This character is often referred to as a null character, and it has a special role in many programming languages, including VBA.
In the realm of C or C++, this is the character that marks the end of a string. While VBA doesn't use null-terminated strings (like C does), vbNullChar
still comes into play in certain scenarios, such as when you're making API calls to DLLs written in C or C++.
vbNullChar vs. vbNullString
In contrast to the vbNullString
constant, vbNullChar
is not an empty string but a character (i.e., a String with a length of one).
Conceptual Differences
vbNullChar
is a character constant that represents a character with an ASCII value of zero. It is an actual character, albeit invisible, and is sometimes treated as a string terminator in languages like C and C++.vbNullString
, on the other hand, is a string constant that represents a zero-length string. It is not an empty string (""
) but a string that doesn't exist. It is a unique data type in VBA used to denote the absence of a string.
Usage Differences
vbNullChar
is mostly used when making API calls to DLLs written in C or C++, where functions expect null-terminated strings. VBA automatically appendsvbNullChar
at the end of strings when passing them to such functions.vbNullString
is used in various scenarios in VBA, such as when you want to differentiate between an uninitialized string and an empty string. It's also useful when you want to declare a string that doesn't allocate any memory.
While vbNullChar
and vbNullString
might seem similar due to their representation of nothingness, they are fundamentally different. Understanding these differences can help you better handle various scenarios in VBA, such as API interaction, string manipulation, and string initialization.
Null Character and API Calls
In many programming languages, such as C and C++, strings are represented as a series of characters ending with a null character (\0
in C/C++ syntax), which has an ASCII value of 0. This null character acts as a sentinel value, indicating the end of the string. This kind of string is often referred to as a null-terminated string.
VBA, however, does not use null-terminated strings. Instead, strings in VBA are stored with their length, and there is no need for a special character to denote the end of the string. UPDATED [2023-11-22]: VBA strings are, in fact, null-terminated, as Ben Clothier correctly points out in the comments below. Unlike C/C++, though, VBA is less reliant on the null terminator as the stored string length allows for more efficient retrieval. I've retained (but struck through) the original article text to provide context for Ben's comments.
VBA, however, does not generally rely on the null character to indicate the end of the string. While VBA strings do have a terminating null character (likely to ease compatibility with C/C++), they are also stored with their length which is a more efficient way to retrieve them from memory than iterating through looking for the null character.
As a refresher, here's how strings are stored in VBA:
Here's how a VBA string is stored in memory:
- 4-byte length prefix: stores the length of the string
- Variable number of two-byte Unicode characters: the string's actual value
- 2-byte NULL termination character: two 0 bytes
The VBA string variable is a pointer to the first Unicode character of the string's actual value.
However, when you're interacting with functions from the Windows API or other DLLs written in C/C++, you may need to pass strings to these functions. These functions often expect strings to be null-terminated, as that's the convention in C/C++.
Fortunately, VBA handles this for you. When you pass a string from VBA to a C/C++ function, VBA automatically appends a null character (vbNullChar
in VBA, equivalent to \0
in C/C++) to the end of the string. This makes the string compatible with the function's expectations.
The caveat here is that you need to ensure there is enough space in the string buffer for this extra character. A buffer is a region of physical memory storage used to temporarily store data while it's being moved from one place to another. If the buffer allocated for the string is exactly the right size for the string data but does not account for the null character, the null character may end up overwriting some other piece of memory, leading to unpredictable behavior or errors in your program.
This can be especially significant when you're working with fixed-size buffers, which are often used in API calls. If you declare a buffer with a size of 100 characters and then try to put a 100-character string into it, there's no room left for the null character. In such cases, you should ensure your buffer size is n + 1
, where n
is the maximum size of the string data, to accommodate the null character.
In summary, when passing strings from VBA to C/C++ functions, be aware that VBA will automatically append a null character to the end of your string, and ensure there is enough space in your buffers to accommodate this extra character.
Null Character in Practice
In VBA, there are fewer use cases for vbNullChar
than for vbNullString
.
However, it can be useful in some scenarios.
String Parsing and Manipulation
For example, vbNullChar
can be used to scan a string for embedded null characters, which can occur when dealing with data from files or API calls.
Dim Parts() As String, MyString As String
MyString = "part1" & vbNullChar & "part2" & vbNullChar & "part3"
Parts = Split(MyString, vbNullChar)
Debug.Print UBound(Parts), Parts(0), Parts(1), Parts(2)
Writing Null Characters to a File
There might be occasions where you need to write a null character to a file. This could be the case when dealing with binary files or specific file formats. This example writes a string containing a null character to a binary file.
Open Environ("tmp") & "\test.txt" For Binary As #1
Put #1, , "Hello" & vbNullChar & "World"
Close #1
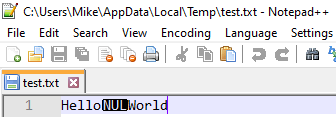
Remember, vbNullChar
represents a character with an ASCII value of zero, so its usage can extend to any situation where such a character is required or useful. However, these situations are relatively rare in day-to-day VBA programming outside of API calls.
Conclusion
While vbNullChar
might seem like an obscure VBA constant, understanding it can help demystify certain aspects of VBA, especially when dealing with API calls or string manipulation. Remember, this "invisible" character is an actual character, and it's treated in a special way by many functions and APIs. Be aware of its presence, and you'll be better equipped to write robust and reliable VBA code.
Acknowledgements
- Portions of this article's body generated with the help of ChatGPT
- One or more code samples generated with the help of ChatGPT
- Cover image created with Microsoft Designer