The vbNullString Constant in VBA
What is the vbNullString constant in VBA and how does it differ from a simple empty string ("")?
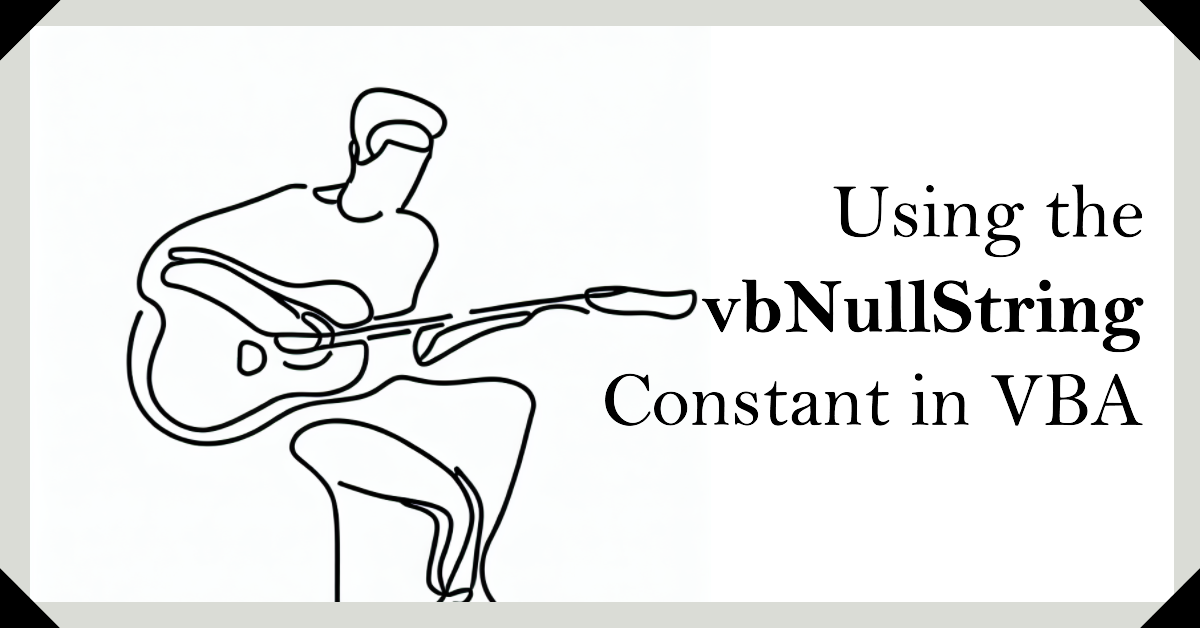
vbNullString is one of many ways to express the concept of nothingness in VBA. For the others, check out An Article About Nothing.
vbNullString
According to section 6.1.2.2–Constants Module–of the VBA Language Specification, the constant vbNullString
is:
An implementation-defined String value representing a null string pointer
What's a Null String Pointer?
The concept is likely unfamiliar because we don't deal with pointers directly in VBA too often.
The best explanations for null pointers come from C/C++ documentation. Those two languages are heavily reliant on pointers (which are nothing more than variables that "point" to addresses in memory). This article has a good working definition of null pointers in the context of C:
In C programming language, a variable that can point to or store the address of another variable is known as pointer. And a null pointer is a pointer which points at nothing.
As far as I can tell, there is no technical difference between a "null pointer" and a "null string pointer". Both terms refer to a variable that does not point to any memory address at all.
However, it's important not to confuse a "null string pointer" with a "null-terminated string." Those are two very different things and getting them mixed up in the context of API calls can lead to hard crashes in VBA.
What's a Null-Terminated String?
A null-terminated string is an array of characters up to and including the NULL character. Here's a good definition from the same article linked above:
In C language string is an array of char type. It stores each of the characters in a memory space of 1 byte. And each array is terminated with ‘\0’ or null character. It is also referred as NUL and is different than NULL or Null Pointer. ...
[I]n C, there are no strings. There are only arrays of characters instead. In C, arrays don't carry length information. So it would be impossible to determine the length of the string by just examining the array. Instead, functions likestrlen
read a string character by character until \0 is encountered in memory. This is why your program might crash or have improper behaviour if you don't NUL terminate the string.
Null String Pointers vs. Null-Terminated Strings in VBA
A String
in VBA is equivalent to the BSTR type in C/C++:
A BSTR (Basic string or binary string) is a string data type that is used by COM, Automation, and Interop functions.
...
A BSTR is a composite data type that consists of a length prefix, a data string, and a terminator.
Every string in VBA is a "null-terminated string" with two exceptions:
- an unitialized
String
variable - the
vbNullString
constant
To demonstrate this, we can use the undocumented StrPtr (string pointer) and VarPtr (variable pointer) functions. The StrPtr function returns the pointer to the actual string data, while the VarPtr function returns a pointer to the variable itself.
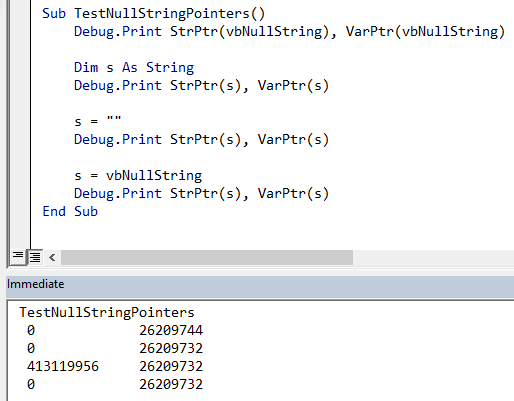
A few notes about the above test code and results:
- StrPtr() returns 0 for the
vbNullString
constant, an unitializedString
variable, and a String variable explicitly set tovbNullString
- The
vbNullString
constant itself gets loaded into memory (VarPtr(vbNullString)
=>26209744
) VarPtr(s)
returns the same value (26209732
) even as the contents of variables
change- When
s
is set to""
, it is no longer a null string pointer (StrPtr(s)
=>413119956
)
Why Should We Care?
We're VBA developers. If we wanted to mess around with pointers and take five times as long to do everything we could just become C programmers. So why should we care about vbNullString, null string pointers, and null-terminated strings?
Because VBA and C/C++ are more closely related than you probably realize.
In fact, I would argue that VBA is as much like C/C++ as it is like VB.NET. While VBA and VB.NET share similar syntax and pseudo-compile to device-independent intermediate code, they are fundamentally different languages under the hood. VBA–like C and C++–is a COM-based language that relies on reference counting for memory management.
It's this shared style of memory management between VBA and C/C++ that makes it worth understanding all this.
VBA may hide the low-level pointer management that is so integral to C/C++ development, but it's still performing those tasks under the hood. The actual pointer manipulation is happening just below the surface of our VBA when we use code like ByRef
, ByVal
, Set ... = Nothing
, CreateObject
, As New
, etc.
Understanding these underlying concepts can help us write more performant VBA along with more reliable API calls.
About Those API Calls...
You need to be a little bit careful about sending null string pointers (i.e., vbNullString
) to certain API calls. Matthew Curland explains in Advanced Visual Basic 6 (p. 359):
The comparison with "" is considered worse than the comparison with vbNullString because "" occupies six bytes of memory; vbNullString is simply a NULL string pointer. The two types are functionally equivalent in VB, but vbNullString is preferred because it doesn't require any memory. You should always use vbNullString instead of "" to clear a string variable. You can occasionally get in trouble with assorted API calls by passing a true NULL instead of an empty string. If you must use an API call that actually requires a string buffer instead of NULL, you should use the StrPtr function to check an incoming string for 0 and replace the zero with "". StrPtr is the only way to differentiate between vbNullString and "".If StrPtr(strData) = 0 Then strData = ""
Additional Reading
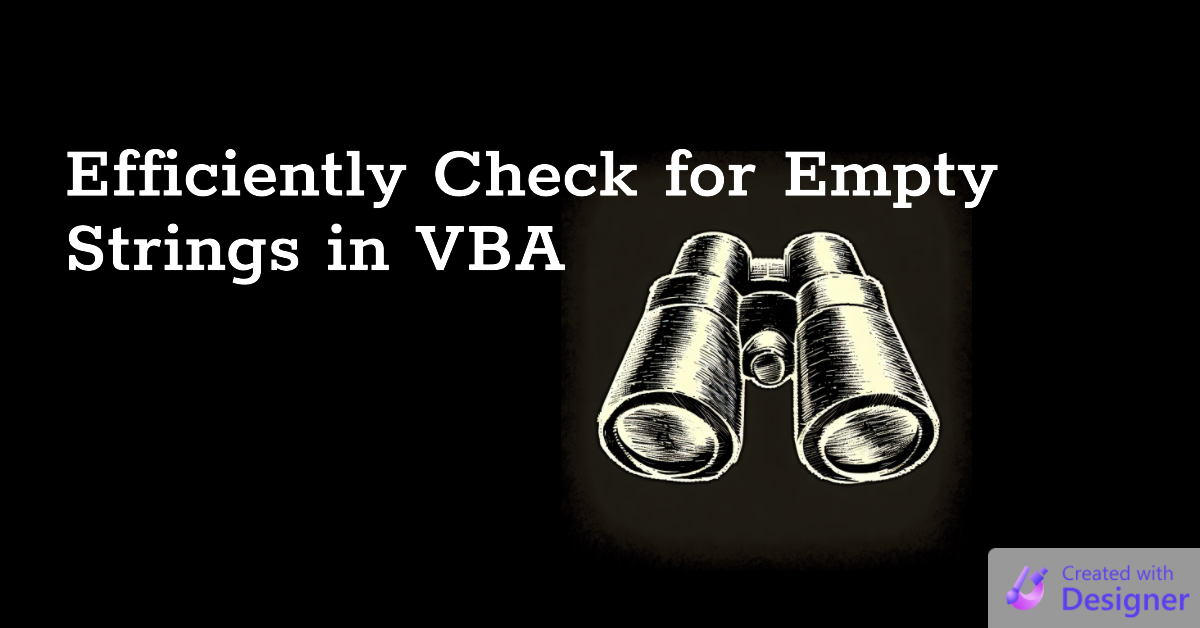
Cover image created with Microsoft Designer