My File System Variable Naming Convention for VBA
"SourcePath" may be one of the worst variable names ever written. Is it the full path to a source file? Is it the folder where my source files are located?
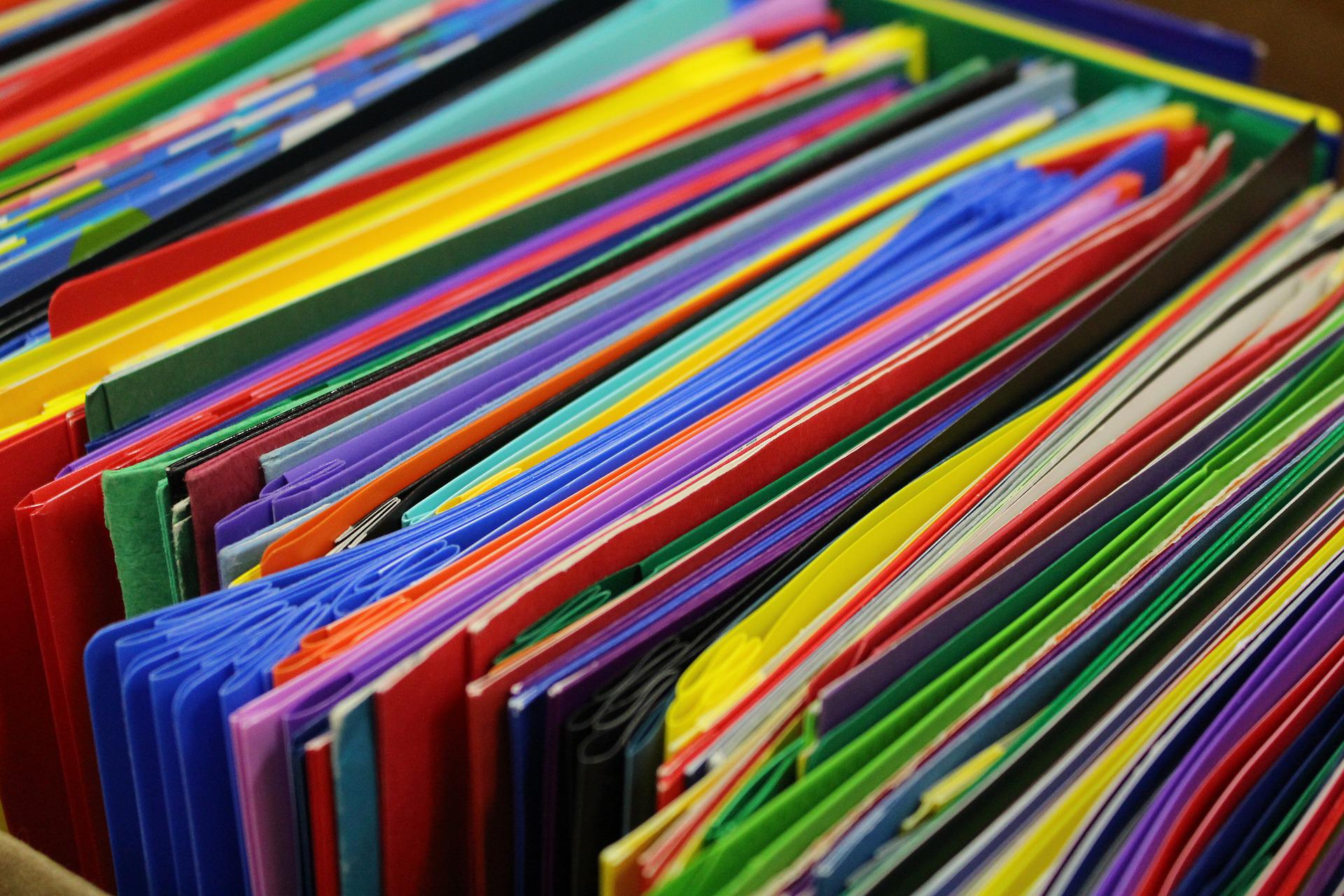
If you're not familiar with his article, Making Wrong Code Look Wrong, take a few minutes to read Joel Spolsky's history of/homage to/diatribe on the "Apps Hungarian" naming convention that eventually got misinterpreted into the abomination that is "System Hungarian."
"Look for coding conventions that make wrong code look wrong. Getting the right information collocated all together in the same place on screen in your code lets you see certain types of problems and fix them right away."
Apps Hungarian vs. Systems Hungarian
Here's the basic difference between the original "Apps Hungarian" and its worse-than-useless mutation, "Systems Hungarian":
- In Apps Hungarian, prefixes indicate the kind of value stored in a variable
- In Systems Hungarian, prefixes indicate the data type of a variable (e.g.,
strMyString
)
Systems Hungarian is useless, because the compiler already handles type checking for us. The value of Apps Hungarian is that it provides additional information about a variable beyond simply its type. Here's another excerpt from Joel's article:
In Word, I’m told, you see a lot of xl and xw, where xl means “horizontal coordinates relative to the layout” and xw means “horizontal coordinates relative to the window.” Both ints. Not interchangeable. In both apps you see a lot of cb meaning “count of bytes.” Yep, it’s an int again, but you know so much more about it just by looking at the variable name. It’s a count of bytes: a buffer size. And if you see xl = cb, well, blow the Bad Code Whistle, that is obviously wrong code, because even though xl and cb are both integers, it’s completely crazy to set a horizontal offset in pixels to a count of bytes.
Folders and Files and Extensions, Oh My!
For a long time, I regularly used the word "Path" in String variables that stored the path to something, for example SourcePath
.
In my opinion, SourcePath
may be one of the worst variable names ever written. Why? Because how are you supposed to know what it's referring to? It's hopelessly ambiguous. Is it the full path to a source file? Is it the folder where my source files are located?
Both of the following lines of code look like they could be logically correct:
SourcePath = "C:\Temp\"
SourcePath = "C:\Temp\MyTempFile.txt"
Or what if I had a function definition that looked like this:
Sub ImportAnalyses(SourcePath As String)
Pop quiz: is that routine looking for the full path to a single file or the path to a folder where the function will ingest all the files it finds there? The SourcePath
argument name provides no clues.
The G&B File System Naming Convention
At Grandjean & Braverman, I've implemented the following naming convention to help make wrong code look wrong when it comes to dealing with files and folders:
File System Variable Names
fp
prefix: Full path; e.g.,C:\Users\Mike\info.txt
or\\dc01\syse\report.pdf
fn
prefix: File name with extension; e.g.,info.txt
orreport.pdf
fno
prefix: File name only; no extension; e.g.,info
orreport
fe
prefix: File extension only; e.g.,txt
orpdf
fo
prefix: Folder only; e.g.,C:\Users\Mike
or\\dc01\syse
Usage
Let's revisit our examples from earlier using this naming convention:
foSource = "C:\Temp\"
fpSource = "C:\Temp\MyTempFile.txt"
Sub ImportAnalyses(fpSource As String)
'This routine imports a single file
End Sub
Sub ImportAnalyses(foSource As String)
'This routine imports every file in the folder passed
End Sub
Whither the Trailing Backslash
As for whether or not a folder variable includes a trailing backslash, I simply don't worry about that anymore. Instead, I use my PathJoin()
function to combine folders and file names into full paths:
fpSource = PathJoin(foSource, fnSource)
External references

Referenced articles
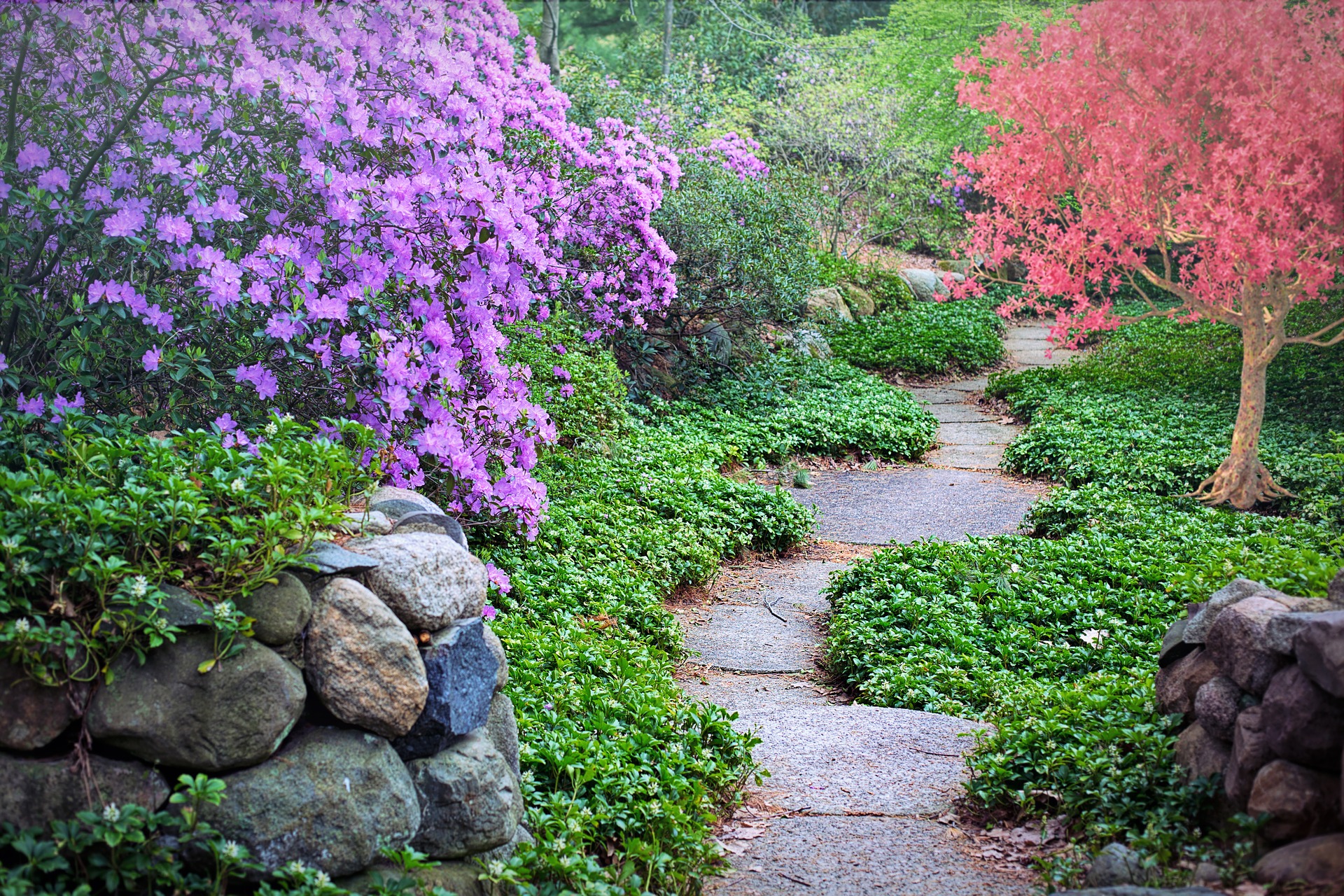