Preventing Line Break After Debug.Print Statement
Every time you call Debug.Print in VBA it outputs to a new line in the Immediate Window. ... But what if it didn't?!?!
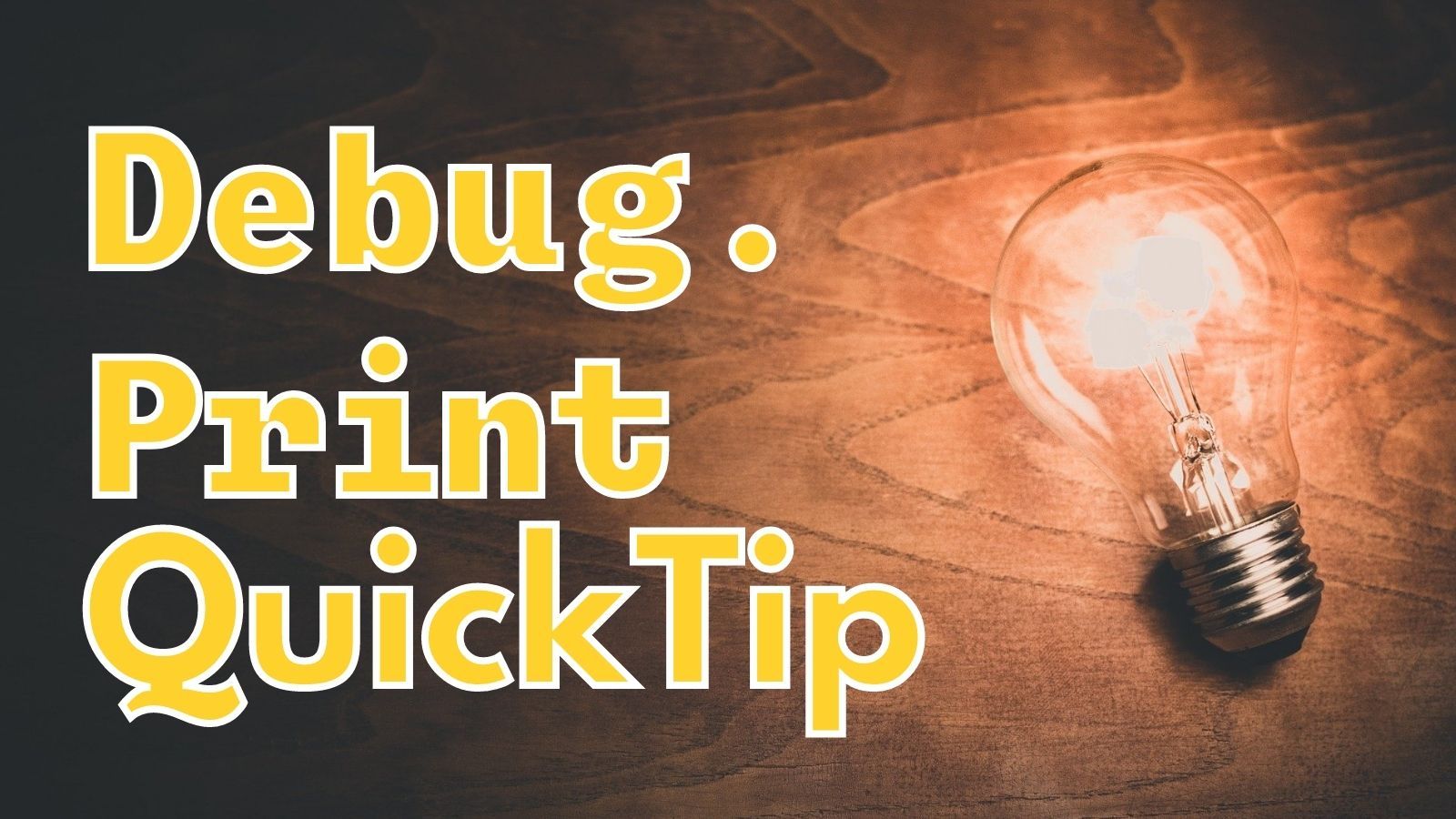
When you execute the Debug.Print statement it outputs the evaluated expression to the immediate window. The cursor then moves to the next line in the window so that each Debug.Print statement will appear on its own line.
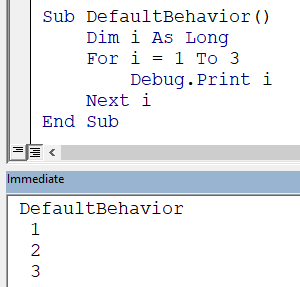
In other words, the default behavior is for Debug.Print to append a newline (specifically a Carriage Return-Line Feed; i.e., vbCrLf
) after outputting the results of the expression.
Appending a Tab Instead of a Newline
If you end your statement with a comma, then Debug.Print appends a Tab character instead of a newline.
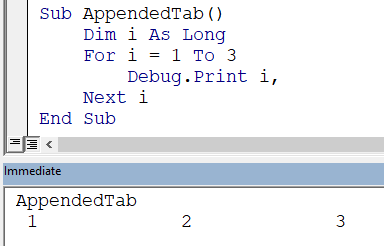
Appending Nothing At All
If you end your statement with a semicolon, then Debug.Print will not append anything after its output.
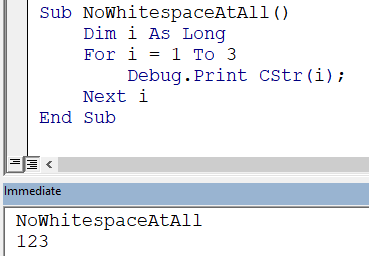
NOTE: In the example code above, I coerced the i
variable to a String
(CStr(i)
) before outputting it because Debug.Print pads numeric values with a leading and trailing space.
Immediate Window Progress Meter
This behavior lets you do interesting things, such as implementing a quick-and-dirty "progress meter" in the immediate window by outputting a single character each time through a loop:
Sub TestPoorMansStatusBar()
Debug.Print "Starting"
Dim i As Long
For i = 1 To 15
Sleep 300
Debug.Print ".";: DoEvents
Next i
Debug.Print "Done"
End Sub
External references
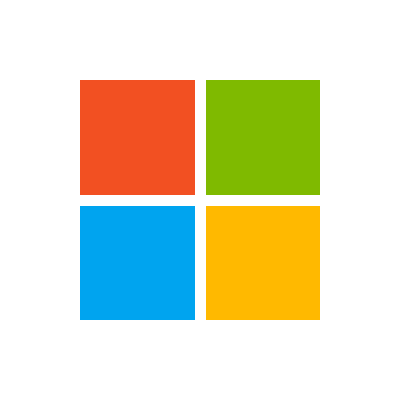
Referenced articles
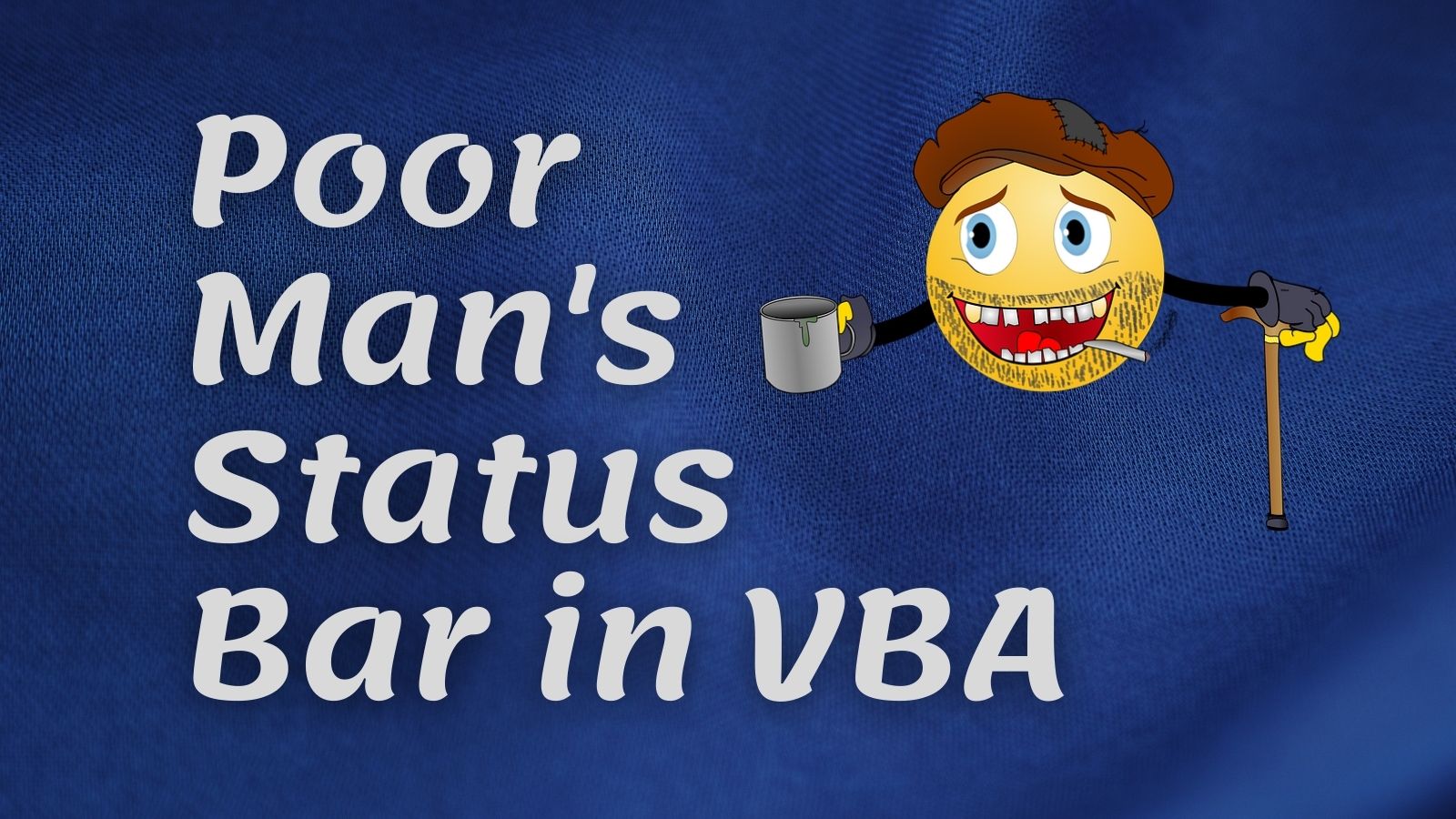