MakeSurePathExists: Using the Windows API to Create Missing Subfolders in VBA
A Windows API function makes verifying (and creating, if necessary) a full folder hierarchy dead simple.
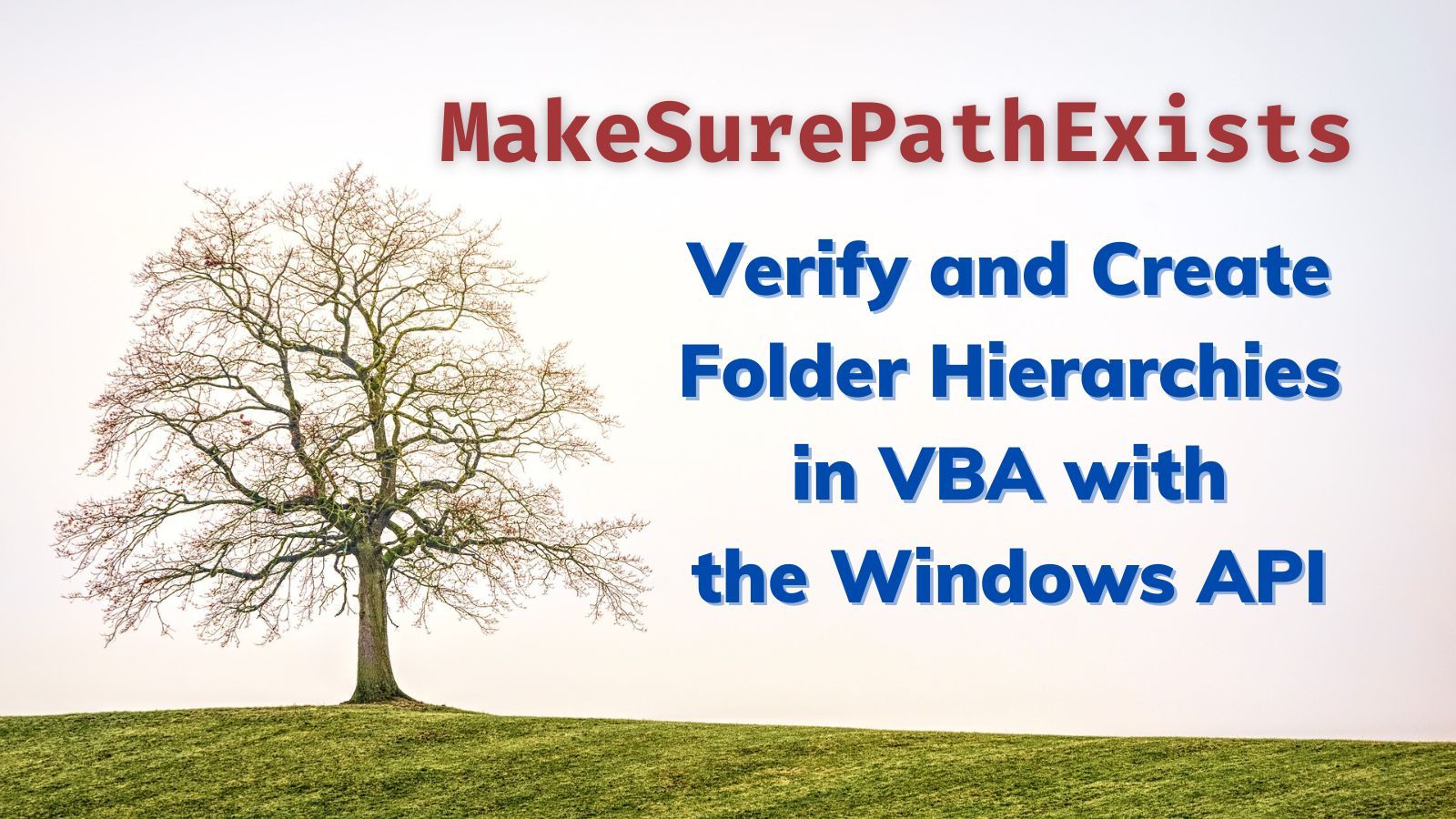
This is part 2 of 3 of a series of articles on creating folder trees in VBA.
I often find the need to verify that an entire subfolder structure exists:
Oftentimes, I'm creating multiple levels of subfolders at a time. For example, I have a folder mapped asG:\Photos
and I need to create the following folder hierarchy,G:\Photos\312\564312\
.
The above quote is from my VerifyFolder()
article. With that function, I used recursion and the FileSystemObject to manually create an arbitrary number of subfolders.
As it turns out, I was reinventing the wheel.
MakeSureDirectoryPathExists API Function
The Windows API includes a function named MakeSureDirectoryPathExists.
Creates all the directories in the specified path, beginning with the root.
Each directory specified is created, if it does not already exist. If only some of the directories are created, the function will return FALSE.
Implementing this API is dead simple. In fact, I don't think we even need to bother with a wrapper function. Let's just alias the function name to something a bit shorter, make sure we include PtrSafe
for 32/64-bit compatibility, and declare it as Public so we only have to declare it once within our project:
'Source: https://nolongerset.com/makesurepathexists/
Public Declare PtrSafe Function MakeSurePathExists _
Lib "Imagehlp" Alias "MakeSureDirectoryPathExists" _
(ByVal DirPath As String) As Boolean
Usage
Just pass it the folder you want it to verify exists. To be on the safe side, you should check the return value to make sure it worked. In practice, I often use this as part of a guard clause:
Sub MyRoutine(MyFolder As String)
If Not MakeSurePathExists(MyFolder) Then Exit Sub
'Do stuff with MyFolder
'...
'...
End Sub
Here's what it looks like running in the Immediate Window:
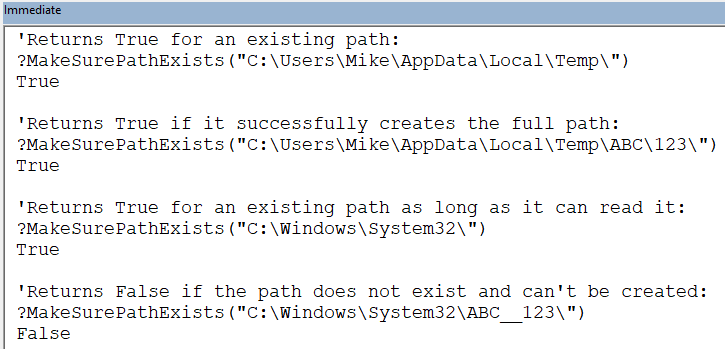
Caveats
Trailing Backslash Required
The function will only create a subfolder if there is a trailing backslash. For instance, in the first example below, the subfolder \ABC\
would be created but not subfolder \123\
:
MakeSurePathExists "C:\ABC\123" 'Creates folder: C:\ABC\
MakeSurePathExists "C:\ABC\123\" 'Creates folders: C:\ABC\123\
Lacks Unicode Support
This function does not support Unicode characters in the path name.
This function does not support Unicode strings. To specify a Unicode path, use the SHCreateDirectoryEx function.
I will implement a Unicode-safe alternative function based on the SHCreateDirectoryEx function in a future article.
Special thanks to Domenico Cozzolino for introducing me to this API function in the comments section of a different article.
External references
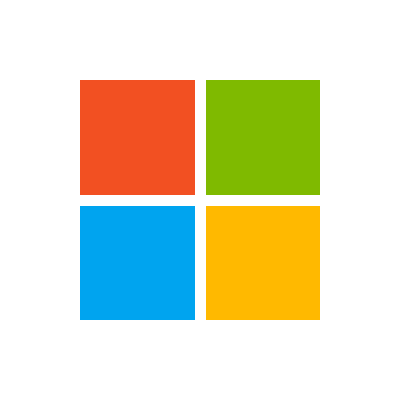
Referenced articles
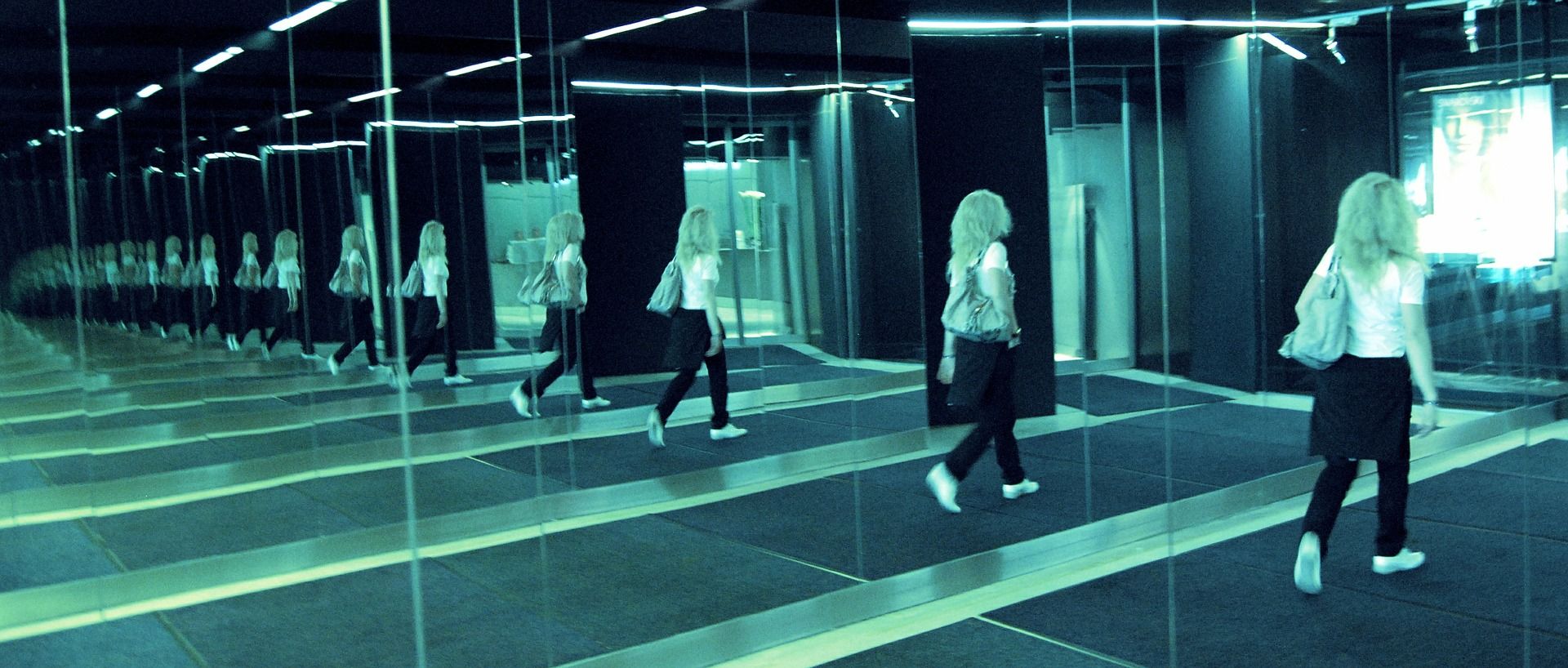