Comment Continuations
Using ellipses to "group" comments separated by intervening lines of code.
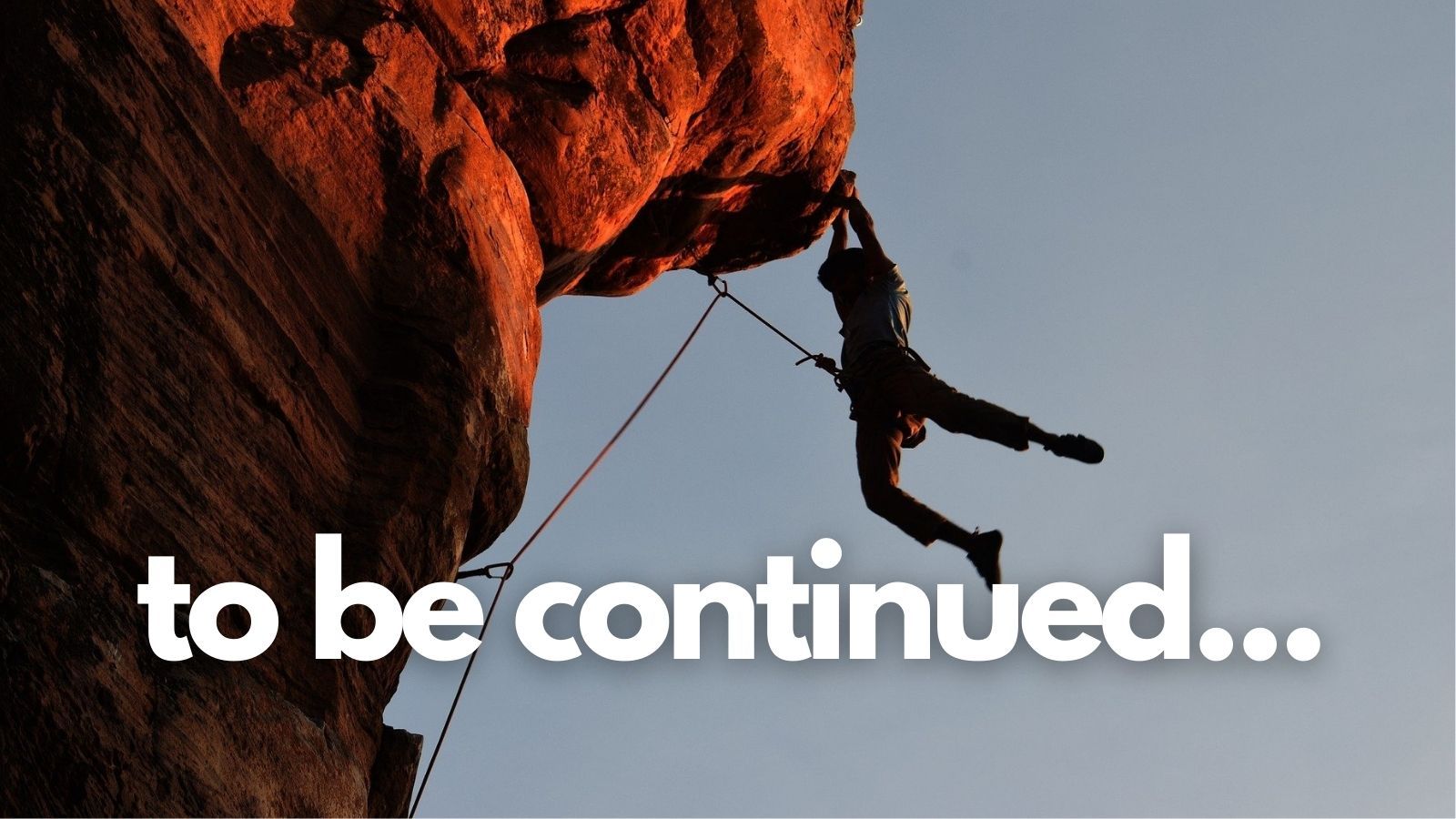
I often want to tie comments from two different lines of code together. I want to have some sort of visual cue on the first line that says, "There's more to this comment."
I'll use a contrived example to make my point for clarity's sake. These examples contain just a few lines of code. In a real program, there are often many more lines in between the start of the comment and its continuation.
The Dilemma
Stack the comments in a block
I could just put the two comments together in a block, but then the second comment is further from the line it describes.
'Pick the first item in list if there is only one,
' otherwise clear the list and force user to choose
If .ListCount = 1 Then
.Value = .ItemData(0)
Else
.Value = Null
End If
Put the comments with their respective lines of code
I could keep each comment on its own line next to the code it's describing. The downside here is that it's less clear that the two comments are related.
If .ListCount = 1 Then
'Pick first item in list if there is only one
.Value = .ItemData(0)
Else
'Otherwise clear the list and force user to choose
.Value = Null
End If
The Solution
Using ellipses to signal intent
The convention I've adopted is to use ellipses to signal to the reader that I intend for the code comment to go along with another comment before or after the current one:
If .ListCount = 1 Then
'Pick first item in list if there is only one...
.Value = .ItemData(0)
Else
'...otherwise clear the list and force user to choose
.Value = Null
End If
Multiple continuations
If I have more than two lines of comments I want to keep together, I will use beginning and ending ellipses for the comments in the middle:
Select Case .ListCount
Case 1
'Pick first item in list if there is only one...
.Value = .ItemData(0)
Case 0
'...raise error if there are no items in the list...
Throw "No items to display."
Case Else
'...otherwise clear the list and force user to choose
.Value = Null
End Select
So What?
As I've written in the past, I strive to maximize the signal to noise ratio in all my projects. With this convention, I am able to clearly articulate my intent without adding much noise. The ellipses provide a strong visual cue that there is more to the story than just the current comment. Since they are performing the same function they do in plain English, there is no need to explain to a reader of my code what their intention is.
And the more our code reads like plain English, the easier it is for others (and us!) to figure out what it's supposed to be doing.