twinBASIC Update: January 16, 2022
Highlights include the CompileIf attribute and IsObject function for generics, an experimental package manager, and 10 Guiding Principles of twinBASIC.
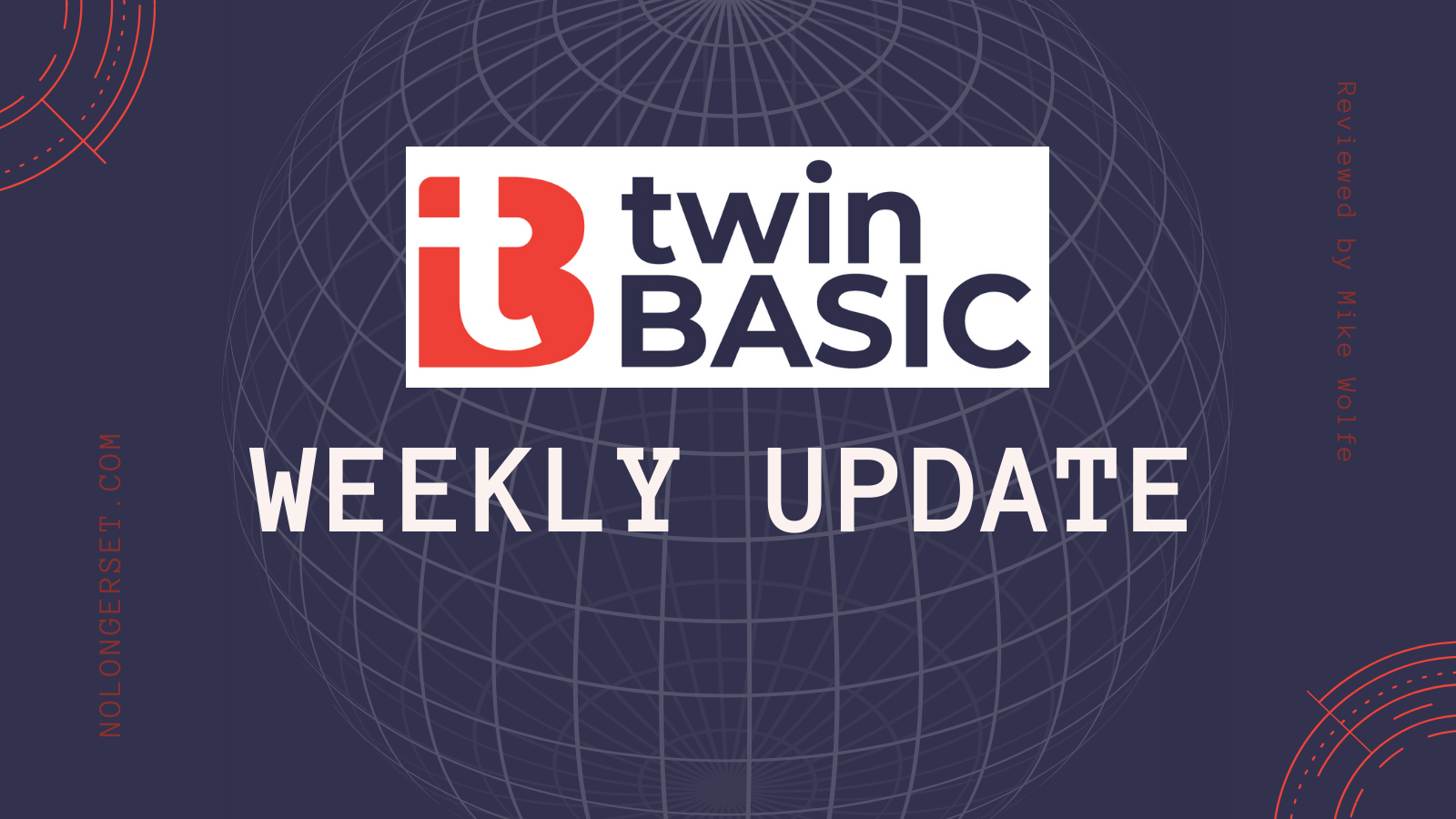
On April 23, 2021, I helped Wayne Phillips introduce the world to twinBASIC at the Access DevCon Vienna conference. I boldly predicted that twinBASIC (along with the Monaco editor) would replace VBA and its outdated development environment by 2025. With that goal in mind, this weekly update is my attempt to keep the project fresh in the minds of the VBA development community.
Every Sunday, I will be providing updates on the status of the project, linking to new articles discussing twinBASIC, and generally trying to increase engagement with the project. If you come across items that should be included here, tweet me @NoLongerSet or email me at mike at nolongerset dot com.
Highlights
Advanced Conditional Compilation
Wayne introduced a new attribute–[CompileIf()]
–for more advanced conditional compilation needs. Along with that attribute comes support for an IsObject()
function that returns–at compile time–whether the variable passed to it is an object or a value type.
This was introduced mainly to support building generic classes, but it opens the door for additional possibilities.
Andrew Mansell provides us with some working sample code demonstrating the two new features:
- The
[CompileIf()]
attribute - The
IsObject()
function
Public Class Tuple(Of T1, T2)
Private _item1 As T1
Private _item2 As T2
Public Property Get Item1() As T1
Return _item1
End Property
[ CompileIf (Not IsObject(Of T1)) ]
Public Property Let Item1(Value As T1)
_item1 = Value
End Property
[ CompileIf (IsObject(Of T1)) ]
Public Property Set Item1(Value As T1)
Set _item1 = Value
End Property
Public Property Get Item2() As T2
Return _item2
End Property
[ CompileIf (Not IsObject(Of T2)) ]
Public Property Let Item2(Value As T2)
_item2 = Value
End Property
[ CompileIf (IsObject(Of T2)) ]
Public Property Set Item2(Value As T2)
Set _item2 = Value
End Property
End Class
Public Class Foo
Public Test As String
End Class
Module Test
Public Sub Main()
Dim x As New Tuple(Of Integer, Double)
x.Item1 = 1
x.Item2 = 3.1415792
Debug.Print x.Item2
Dim y As New Tuple(Of Foo, Foo)
Set y.Item1 = New Foo
Set y.Item2 = New Foo
y.Item2.Test = "Hello Generics!"
Debug.Print y.Item2.Test
End Sub
End Module
As part of this implementation, Wayne has disallowed passing variants as generic types. This makes sense, since generics provide a more compile-time-safe solution to many of the same problems that Variants currently solve.
Experimental Package Manager
On January 13, 2022, Wayne launched a "HIGHLY EXPERIMENTAL" package manager for twinBASIC.
As far as I can tell, most of the details of the package manager have only been discussed in the twinBASIC Discord server. Here are some screenshots:
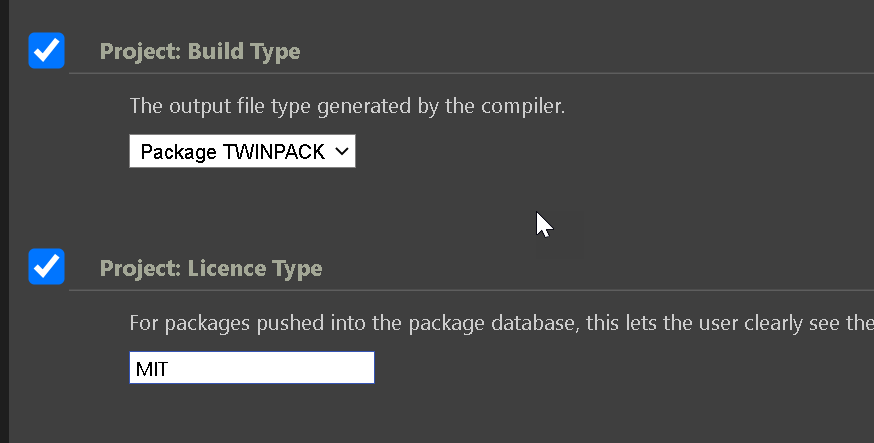
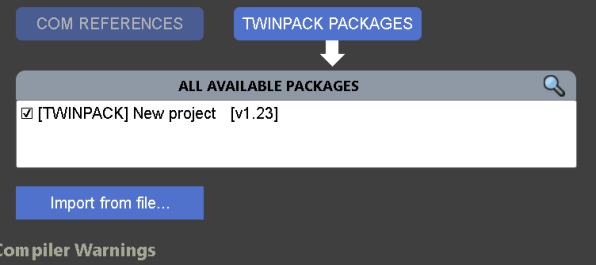
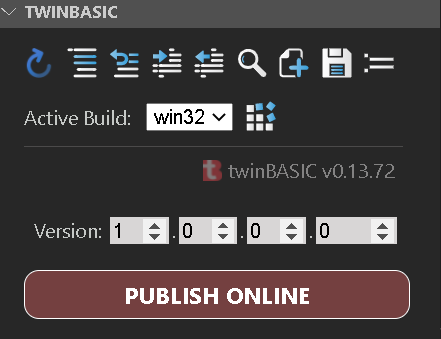
There are several things to note about the current state of the package manager (these are all subject to change):
- All packages include full source code
- All uploaded packages are public
- Wayne has "turned off authentication for now, so at the moment everyone publishes under the same publisher id (TEST-PUBLISHER)"
- Version number supports Major.Minor.Build.Revision so semantic versioning is possible
Planned updates include:
- Support for commercial/private packages
- Curated list of recommended licenses (Apache 2.0, MIT, GPL, etc.)
- Package moderation (to restrict or identify low-quality packages)
- Publisher accounts (I assume)
To test out the new Package feature, press F1 in VSCode then type "twinBASIC: Create new project", press Enter, then choose "Sample 7. Package."
Unit Testing Improvement
When running unit tests, the message accompanying failed assertions now includes both the expected value and the actual value.
Around the Web
twinBASIC Guiding Principles
Ben Clothier has been leading the community through an exercise in distilling the essence of the twinBASIC project.
The 10 Guiding Principles (not quite finalized):
- Backward compatibility trumps everything
- Community is essential
- Solving problems is the point
- Readable code is better than terse code
- Transparency rocks
- Consistency matters
- One way is better than several ways
- Refactoring and testing should be natural
- Documentation is important
- Shipping will be tripping
Be sure to check out Ben's post for the accompanying detailed descriptions that go along with each point.
Array Initialization Syntax
One of the hot topics of discussion this week was if and how to support inline array initialization.
Inline initialization like this,Dim x As Long = 42
, is valid in twinBASIC. If we can do that with simple value types, why not something similar for arrays?
Dim y(2) As Long = 1, 3, 5
Of course, the devil is in the details, and things start to get tricky when you consider support for multi-dimensional arrays and arrays of arrays.
Associative Array Proposal
Associative arrays are a common data structure in many programming languages. There is no such native datatype in VBx. Rather, most VBA programmers rely on the Dictionary object from the Scripting Runtime to provide this feature.
Ben Clothier proposes that this data structure be added as a native datatype in twinBASIC. One of the key benefits of making it a native datatype would be the inclusion of compile-time key name checking. The Scripting Dictionary object relies on keys created at runtime, and so compile-time key name checking is simply not possible in VBx.
Changelog
Here are the updates from the past week. You can also find this information by installing the twinBASIC VS Code extension and clicking on the Changelog tab of the extension page:
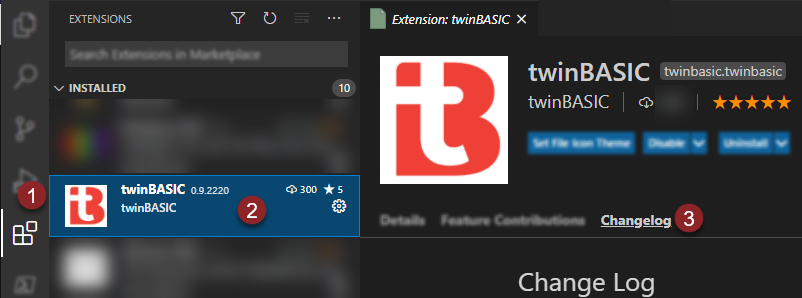
[v0.13.75, 16th January 2022]
- improved: unit test assertions now display the Actual and Expected values in the error information [ https://github.com/WaynePhillipsEA/twinbasic/issues/661 ]
[v0.13.74, 15th January 2022]
- fixed: publisher login/register page not showing for first use
[v0.13.73, 15th January 2022]
- added: Revision and Build version number support in packages
- added: publisher authentication (plus login/register screens) for packages
- fixed: compiler crash global variables with compile-errors [ https://github.com/WaynePhillipsEA/twinbasic/issues/660 ]
- fixed: ParamArray arguments now supported alongside the Implements-Via feature [ https://github.com/WaynePhillipsEA/twinbasic/issues/656 ]
[v0.13.72, 13th January 2022]
- added: HIGHLY EXPERIMENTAL support for packages, and an online package manager
- fixed: renamed 'RGB' parameter to 'RGBA' for RGB_R/RGB_G/RGB_B/RGB_A functions
- fixed: moved MousePointerConstants, MouseButtonConstants and ClipboardConstants enums over to the VBRUN library
- fixed: code prettifier was stumbling on the LHS of augmented assignment operators [ https://github.com/WaynePhillipsEA/twinbasic/issues/651#issuecomment-1010934201 ]
[v0.13.71, 10th January 2022]
- removed: support for passing Variant datatypes as generic specifiers, to help simplify generic implementations [ https://github.com/WaynePhillipsEA/twinbasic/issues/620 ]
- added: generic version of IsObject(Of x), useful for generics conditional compilation
[v0.13.70, 10th January 2022]
- added: CompileIf attribute support, allowing for conditional compilation of procedures based on constant expressions that are evaluated at compile-time (...useful for generics) [ https://github.com/WaynePhillipsEA/twinbasic/issues/620 ]
- added: generic version of VarType(Of x), useful for generics conditional compilation
[v0.13.69, 10th January 2022]
- improved: added an error for 'API declarations in class modules must be Private' to match VBx and provide consitent behaviour [ https://github.com/WaynePhillipsEA/twinbasic/issues/646 ]
- fixed: moved KeyCodeConstants, ColorConstants, SystemColorConstants, FormShowConstants and LoadResConstants over to VBRUN library (previously in VBA) [ https://github.com/WaynePhillipsEA/twinbasic/issues/647 ]
- fixed: moved App and Clipboard classes over to VB library (previously in VBA)
- fixed: type library registration for ActiveX DLLs now populates the 'ThreadingModel' in the registry [ https://github.com/WaynePhillipsEA/twinbasic/issues/609 ]