New Syntax in twinBASIC: Part 3
There's even more new syntax in twinBASIC, including the IsNot operator, initializing on declare, and augmented assignment operators.
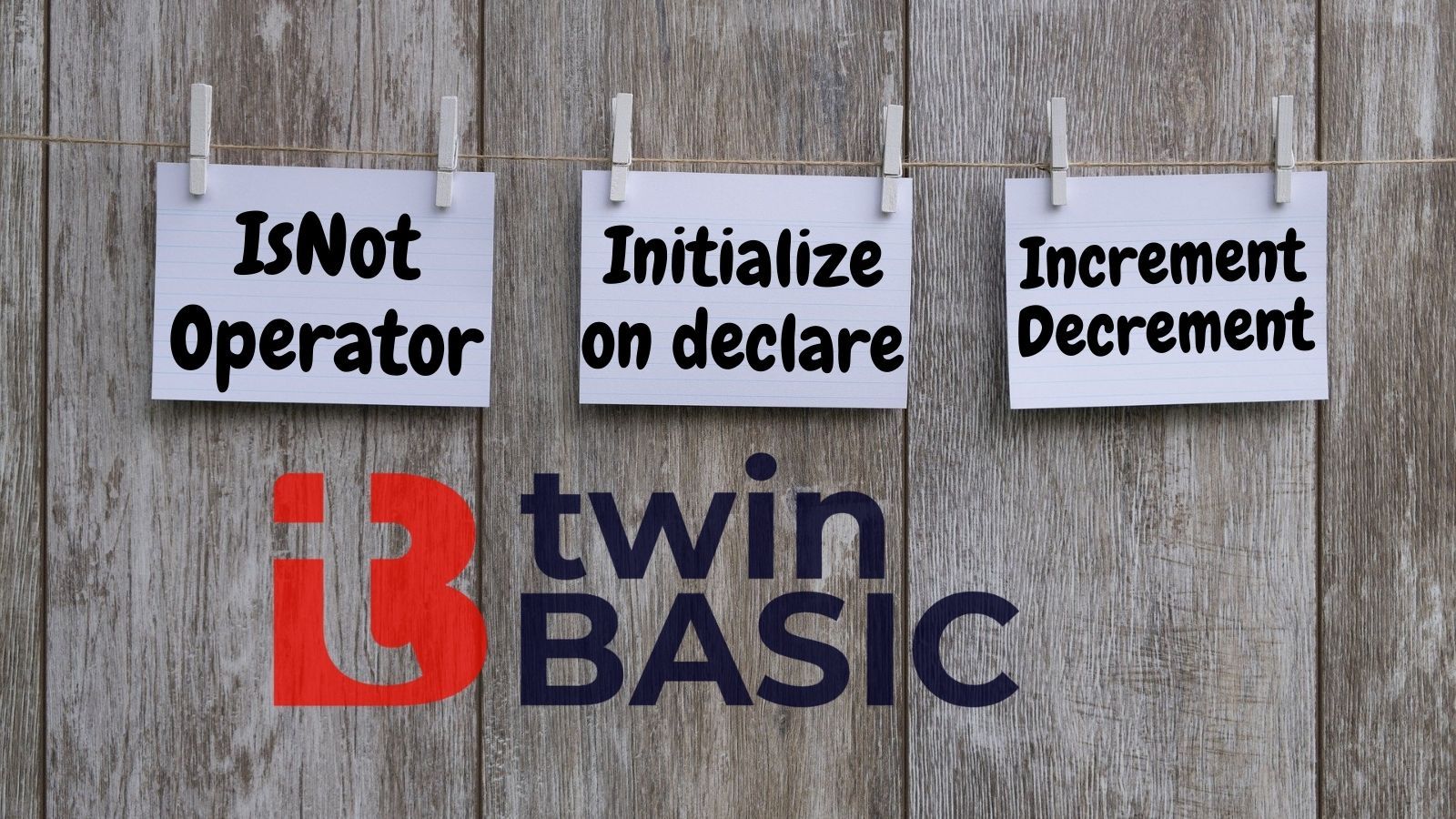
To learn more about twinBASIC, join me at this year's virtual Access DevCon where I will be presenting this exciting new project from vbWatchdog creator, Wayne Phillips.
New Syntax in twinBASIC
twinBASIC introduces several new language features beyond simple VB6 compatibility. Of course, while this syntax and these features are new compared to VB6, most of them are found in VB6's second cousin, twice-removed, VB.NET.
In Part 1, I covered the following new syntax:
- Short-circuiting boolean operators,
AndAlso
andOrElse
- The ternary operator,
If()
- Statements to skip to the next loop operation,
Continue For
, et al.
In Part 2, I covered the following new syntax:
- Parameterized class constructors
- Method overloading
- Return syntax for functions
In this article, I'll cover the following new syntax:
- IsNot operator
- Initialize on declare
- Augmented assignment operators
IsNot Operator
Often in our code we need to check whether an object has been initialized.
If MyObj Is Nothing Then Set MyObj = New clsMyObject
This is easy to read and easy to write.
What's less easy to read and write is checking to see if an object is not initialized.
If Not MyObj Is Nothing Then Set MyObj = New clsMyObject
The meaning is clear, but the phrasing is awkward. The IsNot
operator improves the plain English readability of our code:
If MyObj IsNot Nothing Then Set MyObj = New clsMyObject
Initialize on declare
This new syntax allows you to combine a variable declaration with an initial assignment on a single line. This makes it more convenient and less cluttered than performing the assignment on a separate line.
Instead of this...
Dim i As Integer
i = 1
...or this...
Dim i As Integer: i = 1
...we can now do this (just like in VB.NET):
Dim i As Integer = 1
Augmented assignment operators
You may know these more commonly as increment and decrement operators, but those names only refer to the first two flavors of assignment operators. The following operators are supported:
+=
add in place-=
subtract in place*=
multiply in place/=
divide in place\=
integer division in place^=
exponentiate in place (raise to a power)<<=
bit-shift left in place>>=
bit-shift right in place&=
concatenate string in place
Here's a sampling:
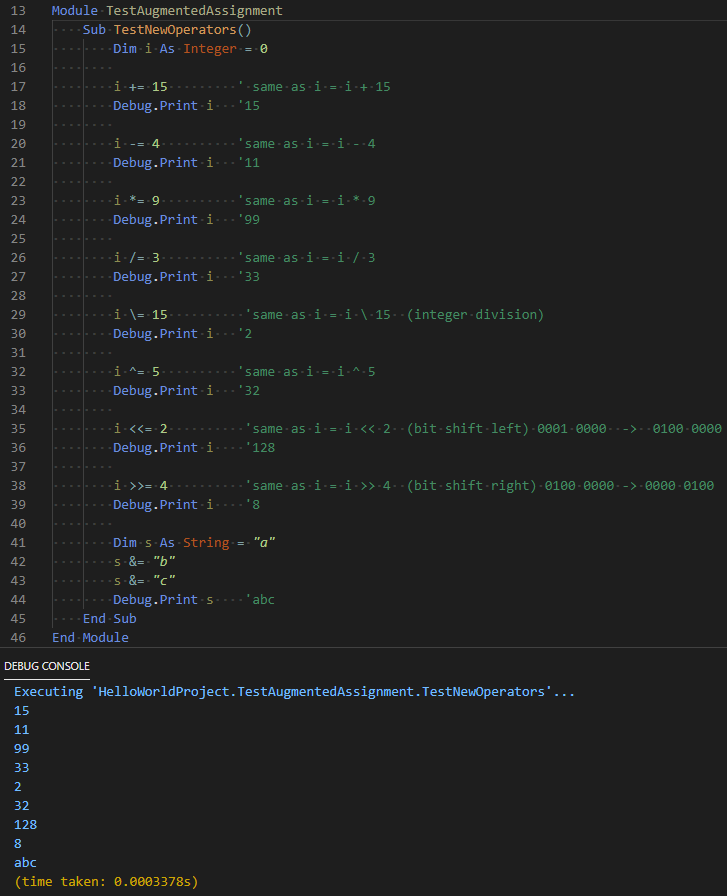
If I'm being completely honest, I'm not sure how I feel about adding this syntax to twinBASIC. I know these assignment operators are available in VB.NET. Actually, that's part of what concerns me.
A short rant about syntactic sugar
What is syntactic sugar?
Syntactic sugar is a syntax shorthand for accomplishing some language operation that is fully supported with a more verbose syntax.
The assignment operators listed above are syntactic sugar, since the exact same operations can be accomplished using more verbose forms. In fact, in the above screenshot, I list the equivalent syntax in the comments.
Most of the other new syntax introduced in twinBASIC has been added to support new operations that were not possible in VBA/VB6, such as Continue For
. And, no, I don't consider GoTo
to be a more verbose syntax for Continue For
; it's an abominable hack born of necessity. In other words, twinBASIC is introducing relatively little syntactic sugar. Overall, the new syntax is high in programming protein.
I worry more about the potential Siren song of syntactic sugar. I don't want twinBASIC to lose the approachability that has made the various BASIC languages so popular over the years.
One of the things that I think makes .NET difficult for new programmers is that there are lots of ways to do identical things; that is, .NET (C# especially) is overflowing with syntactic sugar. Now, I love the nullable type shorthand (int?
is much nicer than Nullable<int>
), but this is exactly the sort of thing that leads to confusion.
Here's a short list of features in C# with multiple ways to accomplish the same thing:
- Implicit typing via
var
- Initializing C# auto-properties
- Nullable types
- Different ways to check for null
- String/string (strings! for crying out loud)
- Etc.
I swear every time Jon Skeet sneezes the world gets some new C# syntax.
-/ end rant /-
Epilogue
I realize after re-reading this very article, that both IsNot
and initializing on declare are examples of syntactic sugar. And I praised each before ranting about augmented assignment operators and the evils of syntactic sugar. So much for consistency.
I'm not about to rewrite the whole thing, so I'll amend my thesis to say that syntactic sugar–like the sugar we eat–should be enjoyed in moderation. I still believe that C# regularly crosses the line into too-much-of-a-good-thing territory with its new syntax. I hope twinBASIC does not follow that same path.