New Syntax in twinBASIC: Part 2
Let's cover some more new syntax in twinBASIC, including parameterized class constructors, method overloading, and return syntax for functions.
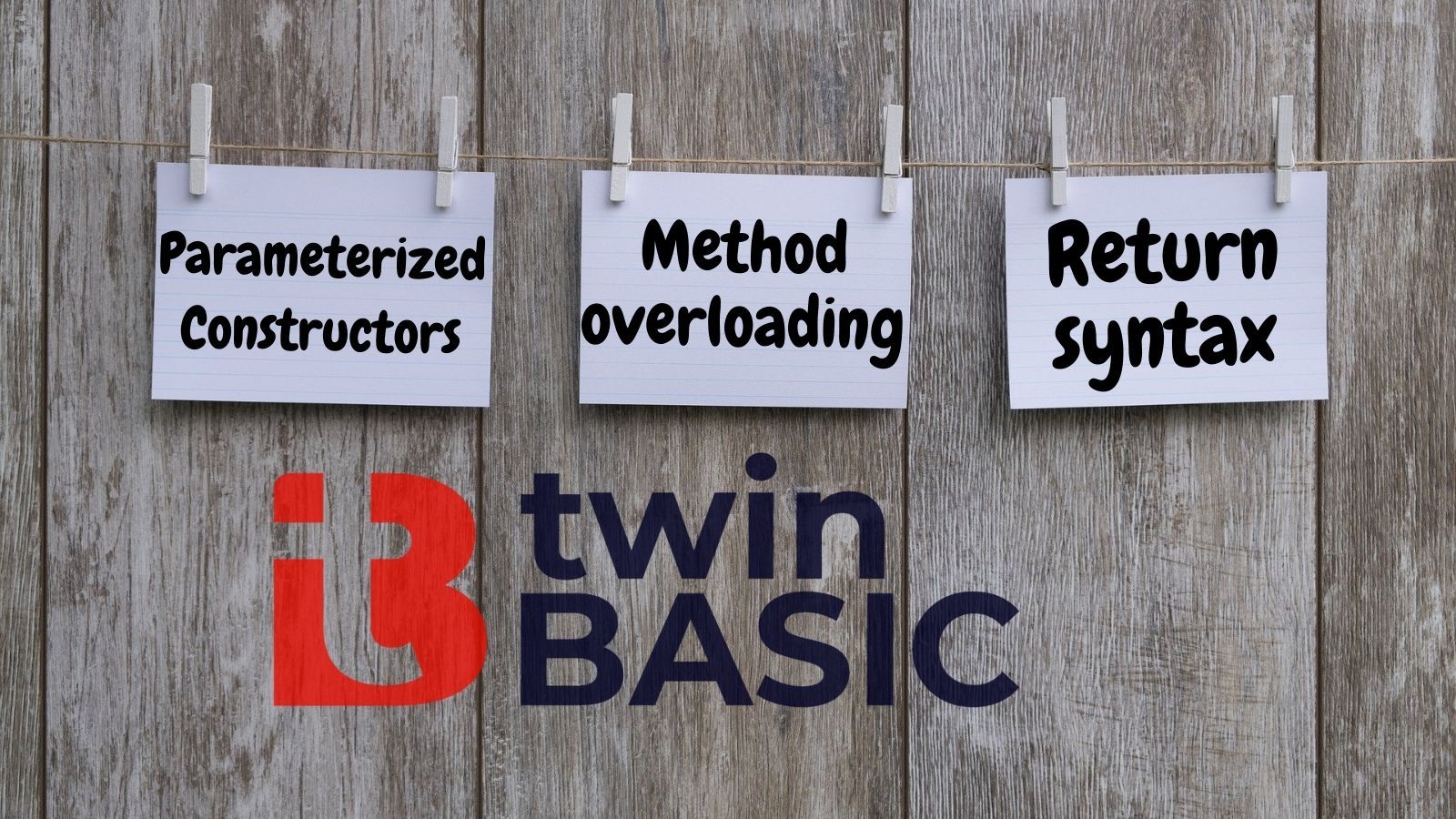
To learn more about twinBASIC, join me at this year's virtual Access DevCon where I will be presenting this exciting new project from vbWatchdog creator, Wayne Phillips.
New Syntax in twinBASIC
twinBASIC introduces several new language features beyond simple VB6 compatibility. Of course, while this syntax and these features are new compared to VB6, most of them are found in VB6's second cousin, twice-removed, VB.NET.
In Part 1, I covered the following new syntax:
- Short-circuiting boolean operators,
AndAlso
andOrElse
- The ternary operator,
If()
- Statements to skip to the next loop operation,
Continue For
, et al.
In this article, I'll cover the following new syntax:
- Parameterized class constructors
- Method overloading
- Return syntax for functions
Parameterized class constructors
One of the features I'm most excited about in twinBASIC is its support for parameterized class constructors. This feature is sorely lacking from VBA, requiring a workaround in the form of factory functions.
The VBA approach
Here's an example of how I handle this situation in VBA using a factory function:
'VBA: the oVehicle class module
Option Compare Database
Option Explicit
Public Make As String
Public Model As String
Public Year As Integer
Function NewVehicleObject(Make As String, _
Model As String, _
Year As Integer) As oVehicle
Set NewVehicleObject = New oVehicle
With NewVehicleObject
.Make = Make
.Model = Model
.Year = Year
End With
End Function
Sub TestDealerLot()
Dim Lot As New Collection 'of oVehicle objects
Lot.Add NewVehicleObject("Ford", "Mustang", 1968)
Lot.Add NewVehicleObject("Dodge", "Challenger", 1970)
Lot.Add NewVehicleObject("Chevy", "Camaro", 1969)
Dim Car As Object 'oVehicle
For Each Car In Lot
Debug.Print Car.Make, Car.Model, Car.Year
Next Car
End Sub
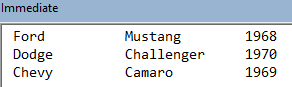
The twinBASIC approach
Now, let's take advantage of twinBASIC's parameterized constructor feature to rewrite the above code. In addition to the familiar Class_Initialize()
method, we can now create public methods named New()
and pass zero or more variables to those methods:
Class oVehicle
Public Make As String
Public Model As String
Public Year As Integer
Public Sub New()
End Sub
Public Sub New(Make As String, _
Model As String, _
Year As Integer)
Me.Make = Make
Me.Model = Model
Me.Year = Year
End Sub
End Class
Module TestParamCtors
Sub TestDealerLot()
Dim Lot As New Collection 'of oVehicle objects
Dim Vehicle As New oVehicle
Set Vehicle = New oVehicle("Ford", "Mustang", 1968): Lot.Add Vehicle
Set Vehicle = New oVehicle("Dodge", "Challenger", 1970): Lot.Add Vehicle
Set Vehicle = New oVehicle("Chevy", "Camaro", 1969): Lot.Add Vehicle
Dim Car As Object 'oVehicle
For Each Car In Lot
'These two lines of code are required while twinBASIC is in preview
Dim Auto As oVehicle
Set Auto = Car
Debug.Print Auto.Make & " " & Auto.Model & " " & Auto.Year
Next Car
End Sub
End Module
Also, notice in the code below how the semantic highlighting makes it clear which Make
/Model
/Year
values go together:
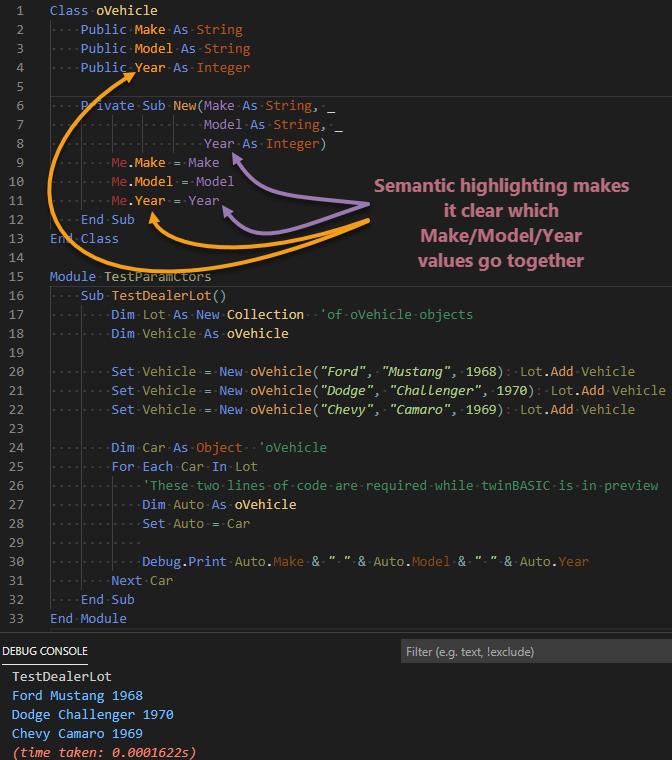
Method overloading
Method overloading allows us to declare the same routine name more than once in the same scope but with a different number and/or type of parameters. How might this be useful? One common scenario is looking up a database record by autonumber ID or by a unique key field.
For example:
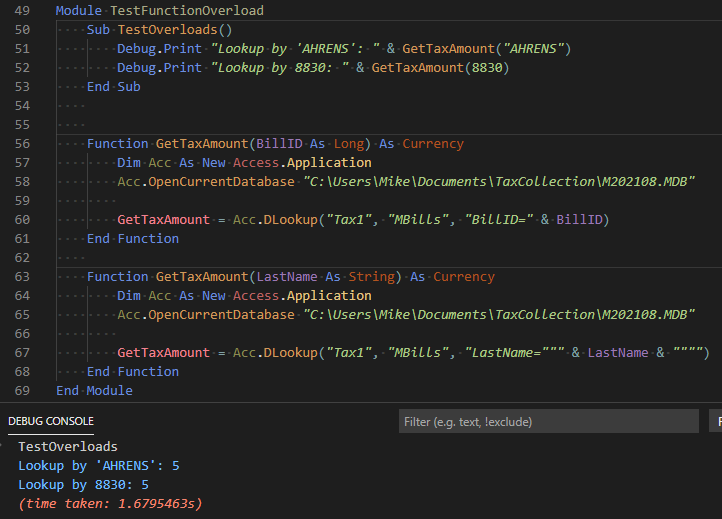
Return syntax for functions
Returning a value from a function by assigning that value to an identifier with the same name as the function felt awkward to me when I first started writing VBA. I think using the Return syntax makes code read more like plain English, which is always a good thing.
Keep in mind, though, that using the Return syntax is like including an implicit Exit Function
call immediately after the Return
line. Thus, any cleanup code you might have in place would need to be called before the Return call.
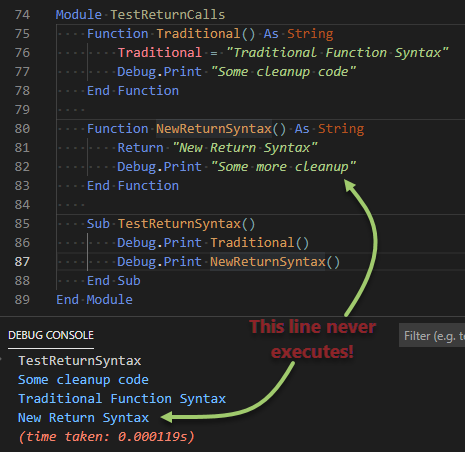
Return
line.There is one last point about the new return syntax. In order to maintain full backwards compatibility with VB6/VBA, you cannot use this new syntax within a procedure that contains GoSub
subroutines. (There's no reason to use those, anyway, so this is not much of a limitation.)
CORRECTION [2021-05-27]: Updated sample code for twinBASIC constructors from Function to Sub. Constructors must be Sub's.