Invalidating the Cache on the Hidden Duplicate Values Form
In this installment, we demonstrate how simple it is to refresh the cache when you use self-healing object variables.
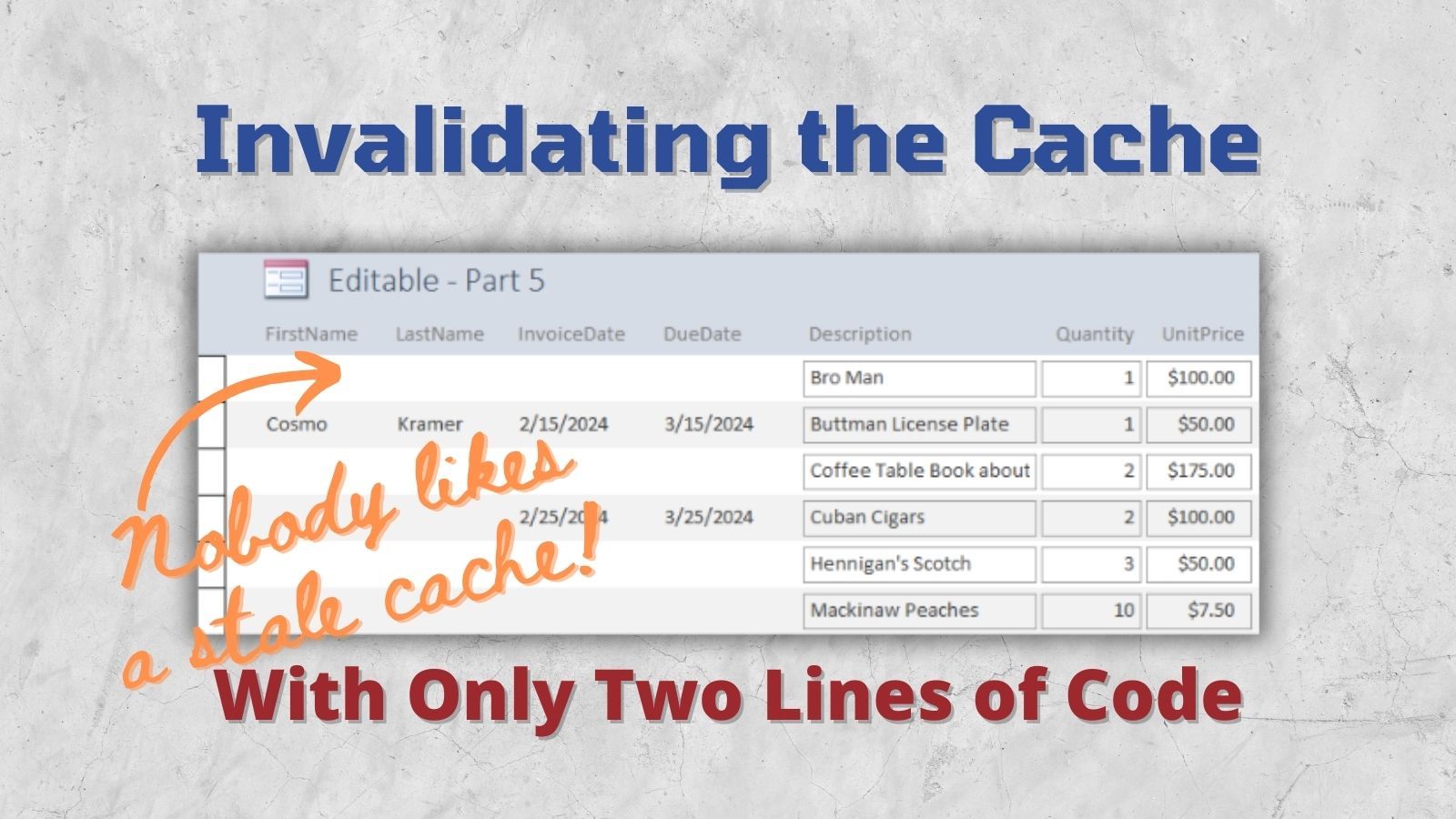
This article is Part 7 of my "Hiding Duplicate Values" Mini-Series.
To follow along with the screenshots and examples, download the sample data from here: Sample Data for the "Hiding Duplicate Values" Series.
Cache invalidation is hard. Like, really hard:

But what is "cache invalidation" and why does it matter for this article series?
Cache Invalidation
As is often the case, Wikipedia has an excellent succinct definition:
Cache invalidation is a process in a computer system whereby entries in a cache are replaced or removed.
The reason caching boosts performance is that it limits the amount of time you spend performing expensive operations, like database access.
But what if the underlying data changes from the time you last cached it? Now the cache is invalid. You need to replace or remove the results of the invalid calculation.
But how do you know if the underlying data changed? To be safe, you could check it every single time your function is called. Of course, then you're right back to where you started, and you don't really have a cache at all.
Instead, you need some way to verify the contents of the cache periodically. "Periodically" could mean:
- At regular intervals (e.g., every five minutes)
- Whenever a record is updated on the current form
- Whenever any change is made to the underlying database (hello, rowversion!)
- Whenever the form is refreshed
- Via an explicit [Refresh] button on the form
- Whenever the form is opened
There is no one correct approach. It all depends on the situation.
Cache invalidation is really hard.
Actually, Cache Invalidation Is Not Hard
Knowing when to invalidate the cache is hard.
Actually invalidating the cache? That can be really easy.
In fact, the cache invalidation function for our code from Part 6 is two lines:
'Invalidate the cache
Private Function ClearCache()
Set this.TopCustomerItems = Nothing
Set this.TopInvoiceItems = Nothing
End Function
That's it. We just set the dictionary objects to nothing.
The next time either function is called, it will start by checking to see if the passed InvoiceID or CustomerID value exists in the respective dictionary object. Seeing that the value does not exist in the dictionary, it will add it back in. The cache will be automatically repopulated without any other work from us. It's one of the key benefits of self-healing object variables.
Clean and simple.