Mailgun: Quick Start Guide for VBA Developers
Sample VBA code and step-by-step instructions for sending a simple test email via the Mailgun email service (no credit card needed).
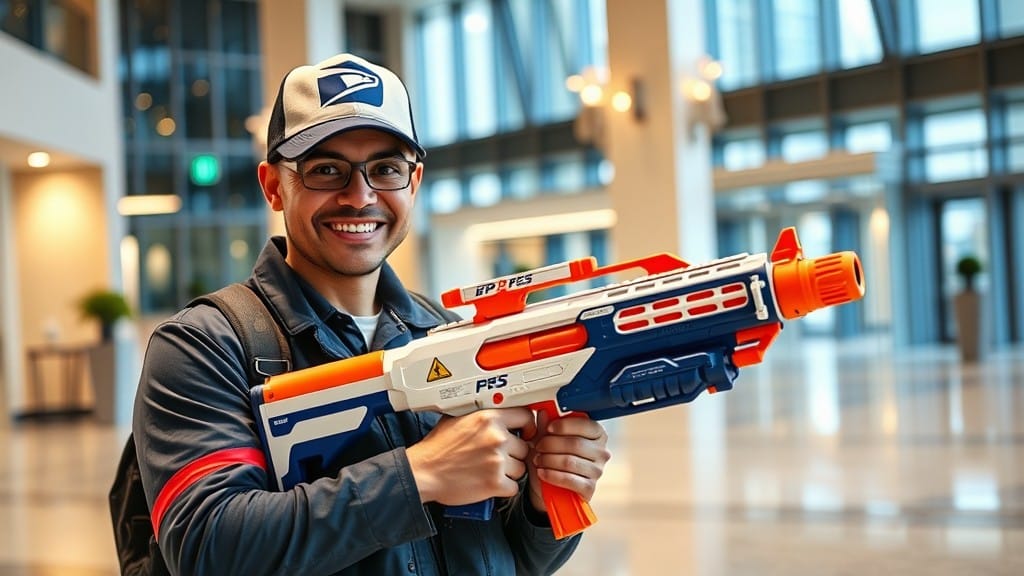
In the words of my teenage daughter, Microsoft is going "full send" on its transition to New Outlook.
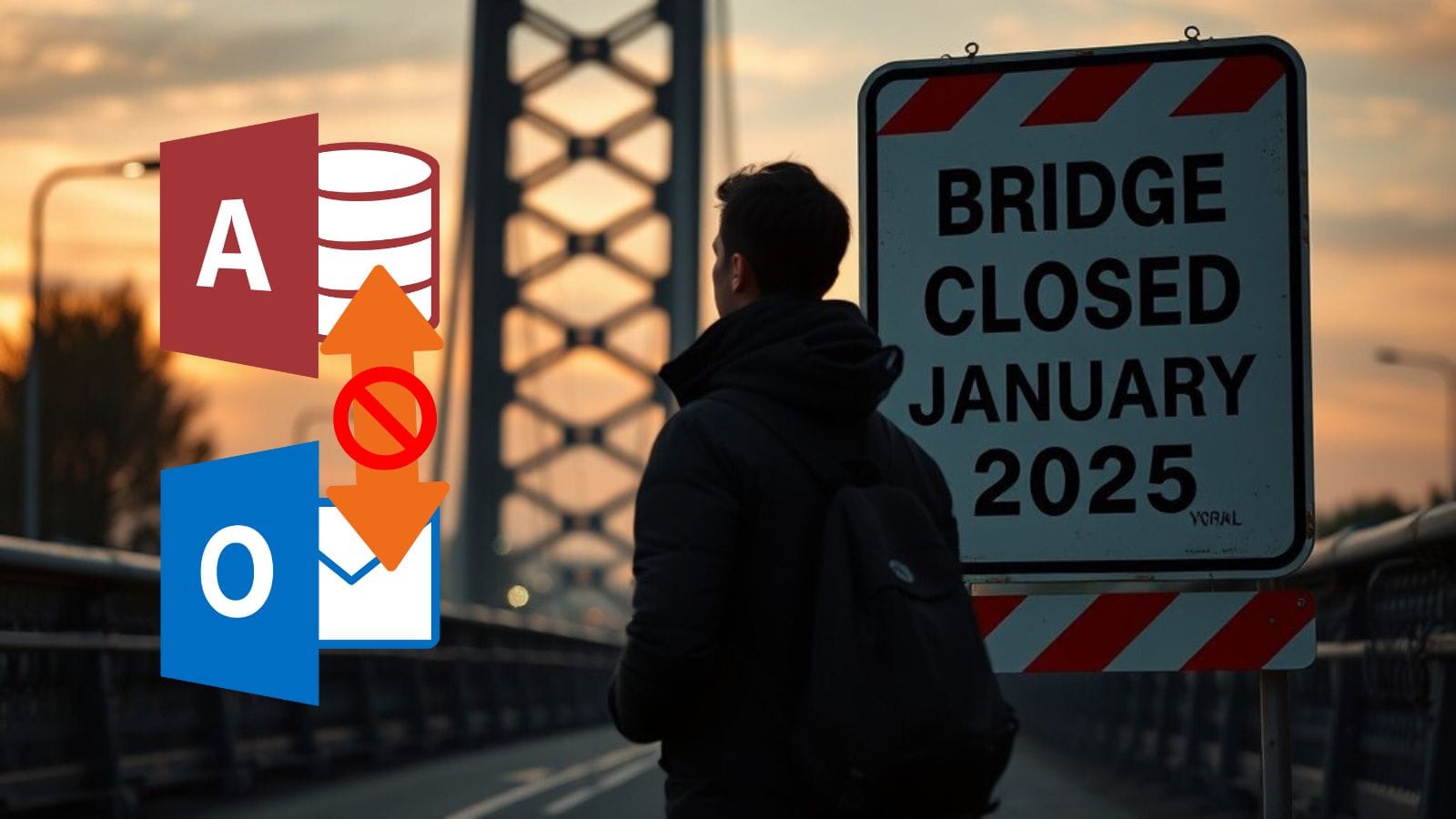
With that in mind, we as Access developers need to start preparing for this transition. There's still time for Microsoft to come up with an official workaround–Classic Outlook will be supported through at least 2029–but it's never too early to start thinking about alternatives. In an earlier article, I floated the idea of using Mailgun as one such COM-free alternative.
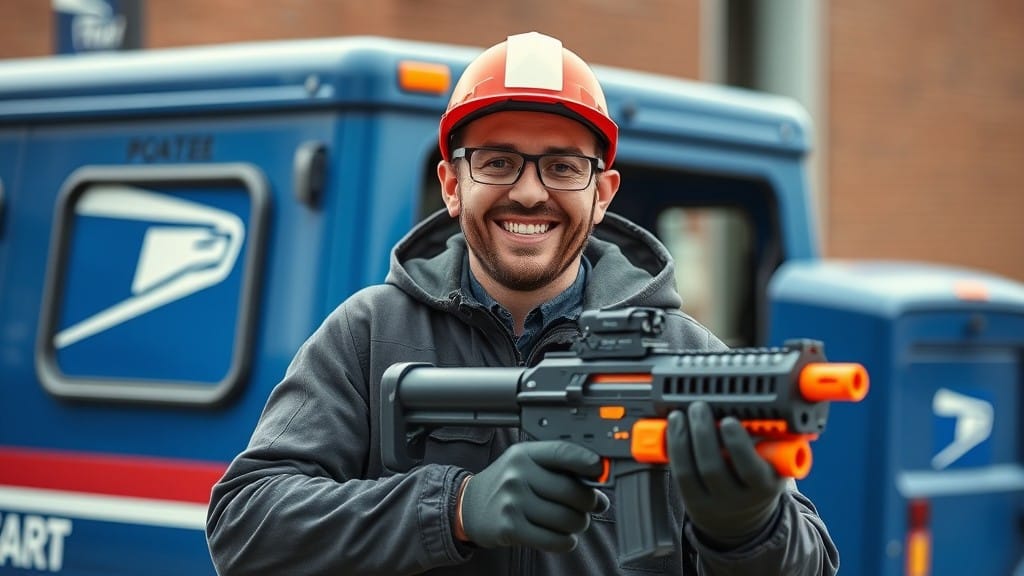
Here's a quick start guide to sending a simple proof-of-concept email with Mailgun via VBA.
Sign Up For a Free Account
Visit mailgun.com and click the [Get Started for Free] button.
As of writing, the sign up screen will default you to a 30-day trial of their $35/mo Foundation plan. You can do that if you want, but be aware that you will need to provide credit card info as part of the signup.
The free tier supports sending of up to 100 emails a day and grants full API access. That's perfect for our initial testing.
You will need two things to sign up for a free account:
- A valid email address
- A mobile phone number
Note that you do not need a credit card to test the free plan.
Getting Started
Upon logging in for the first time, you will be greeted with the following "Get started guide":
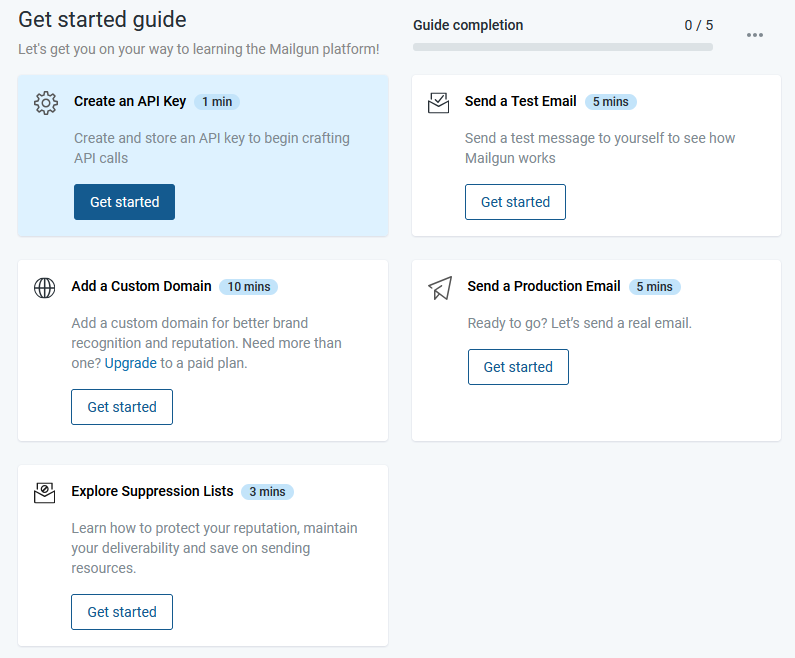
There are five steps:
- Create an API key
- Send a Test Email
- Add a Custom Domain
- Send a Production Email
- Explore Suppression Lists
We will cover the first two steps in this article just to prove the concept and get you some working VBA code.
Create an API Key
API keys are passwords that you use in your code to connect with the Mailgun service.
You can create as many API keys as you want. For security, you'll want to create multiple keys so that if one gets compromised you can delete it without breaking all your other applications. For now, though, we just need to create one key to test the service.
- Click [Create API key]
- Enter a description for the new API key (I used "Initial Test")
- Click [Create Key]
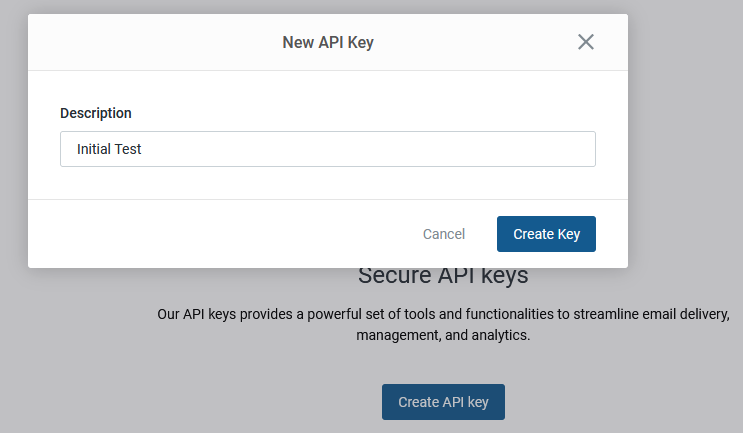
Mailgun only shows the full API key once, so save it somewhere safe. I saved mine in my KeePass database.
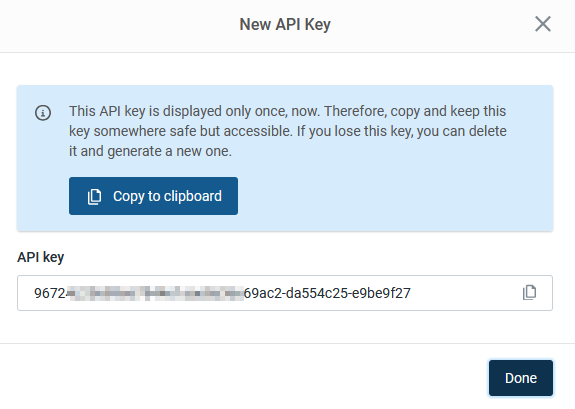
If you do lose the API key, simply delete the key from your account and generate a new one.
All your valid API keys appear in the Mailgun API keys page. For each key, you will see the:
- Key ID: the last 16 hex characters of your full API key
- Description: the description you gave your key when you created it
- Created date: the date you created the key
After creating your first key, click the "Get started" page on the left nav bar to move on to the next steps.
Send a Test Email
There are two ways to get to the page with details for sending your test email:
- Via the "Get Started" Menu
- Click [Get started] under the "Send a Test Email" heading - Via the Main Navigation sidebar
- Navigate to Send > Sending > Overview
Configure an "Authorized Recipient" email address
First, you must set up and verify an "Authorized recipient" email address. This lets us test the service without a credit card while disincentivizing abuse by spammers.
- Enter an email address you control in the "Authorized Recipients" text box and then click on [Save Recipient]
- The recipient email will immediately appear under the [Save Recipient] button along with "Unverified" in orange letters
- Go check your email and click the links to accept test emails from Mailgun
- Refresh the web page and the orange "Unverified" text should be replaced with green "Verified" text
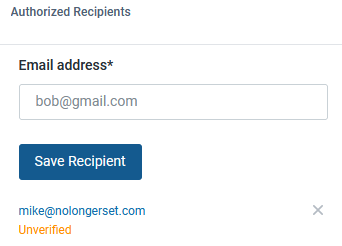
API: Get Your Unique Info
It's hard to believe, but Mailgun does not provide sample VBA code (I know, right?). Don't worry, though. I've got you covered.
Before we get to the sample code, you will need some info that's unique to your account. The easiest way to get that is via the cURL sample:
- Click the [Select] button under the API heading
- Click cURL to show the sample curl code
- Note the URL (box 1) and From Address (box 2) in the screenshot below; you will need to copy and paste those strings into constants in the VBA sample code
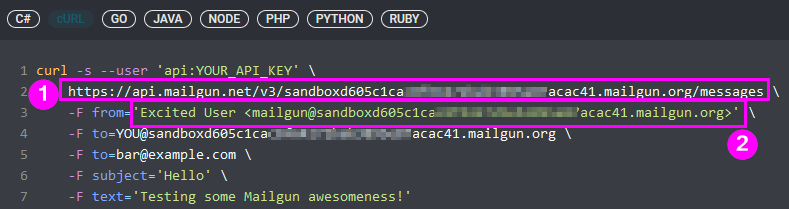
VBA Sample Code: Simple Email Sending Test
Here's some sample code to test the integration.
- Copy and paste the sample code below into your Access application
- Overwrite the
Url
constant with the cURL sample text from Box 1 above - Overwrite the
FromAddress
constant with the cURL sample text from Box 2 above - Run
SendEmailWithMailgun
from the immediate window
If all goes well, you should see a message box that says "Email sent successfully!"
If there are problems, you will get a "Failed to send email" message, along with the specific HTTP error code and response text from the Mailgun server.
Sub SendEmailWithMailgun()
' Constants
Const ApiKey As String = "0123456789abcdef0123456789abcdef-01234567-89abcdef" '<-- REPLACE WITH YOUR INFO
Const Url As String = "https://api.mailgun.net/v3/sandbox0123456789abcdef0123456789abcdef.mailgun.org/messages" '<-- REPLACE WITH YOUR INFO (get from cURL example on mailgun.com)
Const FromAddress As String = "Excited User <mailgun@sandbox0123456789abcdef0123456789abcdef.mailgun.org>" '<-- REPLACE WITH YOUR INFO (get from cURL example on mailgun.com)
Const ToAddress1 As String = "youremail@example.com" '<-- REPLACE WITH YOUR INFO (must be a verified recipient if you are testing with the free plan)
Const Subject As String = "Hello"
Const BodyText As String = "Testing some Mailgun awesomeness!"
' Prepare the POST data
Dim PostData As String
PostData = "from=" & UrlEncode(FromAddress) & _
"&to=" & UrlEncode(ToAddress1) & _
"&subject=" & UrlEncode(Subject) & _
"&text=" & UrlEncode(BodyText)
' Create the HTTP request object
Dim Http As Object
Set Http = CreateObject("MSXML2.XMLHTTP")
' Open the request
Http.Open "POST", Url, False
' Set the authentication header
Http.SetRequestHeader "Authorization", "Basic " & EncodeBase64("api:" & ApiKey)
' Set the content type
Http.SetRequestHeader "Content-Type", "application/x-www-form-urlencoded"
' Send the request with the POST data
Http.Send PostData
' Check the response
If Http.Status = 200 Then
MsgBox "Email sent successfully!", vbInformation
Else
MsgBox "Failed to send email. Status: " & Http.Status & vbCrLf & "Response: " & Http.ResponseText, vbExclamation
End If
' Clean up
Set Http = Nothing
End Sub
' Function to encode data for URL parameters
Function UrlEncode(ByVal Text As String) As String
Dim i As Long
For i = 1 To Len(Text)
Dim Char As String
Char = Mid(Text, i, 1)
Dim Result As String
Select Case Asc(Char)
Case 48 To 57, 65 To 90, 97 To 122 ' 0-9, A-Z, a-z
Result = Result & Char
Case Else
Result = Result & "%" & Hex(Asc(Char))
End Select
Next i
UrlEncode = Result
End Function
' Function to encode a string in Base64 format
Function EncodeBase64(ByVal Text As String) As String
Dim ArrData() As Byte
ArrData = StrConv(Text, vbFromUnicode)
Dim ObjXml As Object
Set ObjXml = CreateObject("MSXML2.DOMDocument")
Dim ObjNode As Object
Set ObjNode = ObjXml.CreateElement("b64")
ObjNode.DataType = "bin.base64"
ObjNode.NodeTypedValue = ArrData
EncodeBase64 = ObjNode.Text
' Clean up
Set ObjNode = Nothing
Set ObjXml = Nothing
End Function
What's Next
In future articles, we will explore more advanced use cases including:
- Multiple To:/CC: email addresses
- Attaching files
- Sending HTML emails
- Setting up custom sending domains
- Supporting multiple customers (i.e., multi-tenant configuration)
Keep an eye on the Email tag for related articles.
Acknowledgements
- One or more code samples generated with the help of ChatGPT
- Cover image generated by FLUX-schnell