Calculating Gift Counts for "The Twelve Days of Christmas": Looping Edition
In the first solution to my recent reader challenge, I use a loop to calculate the cumulative number of gifts given for each day of the "12 Days of Christmas."
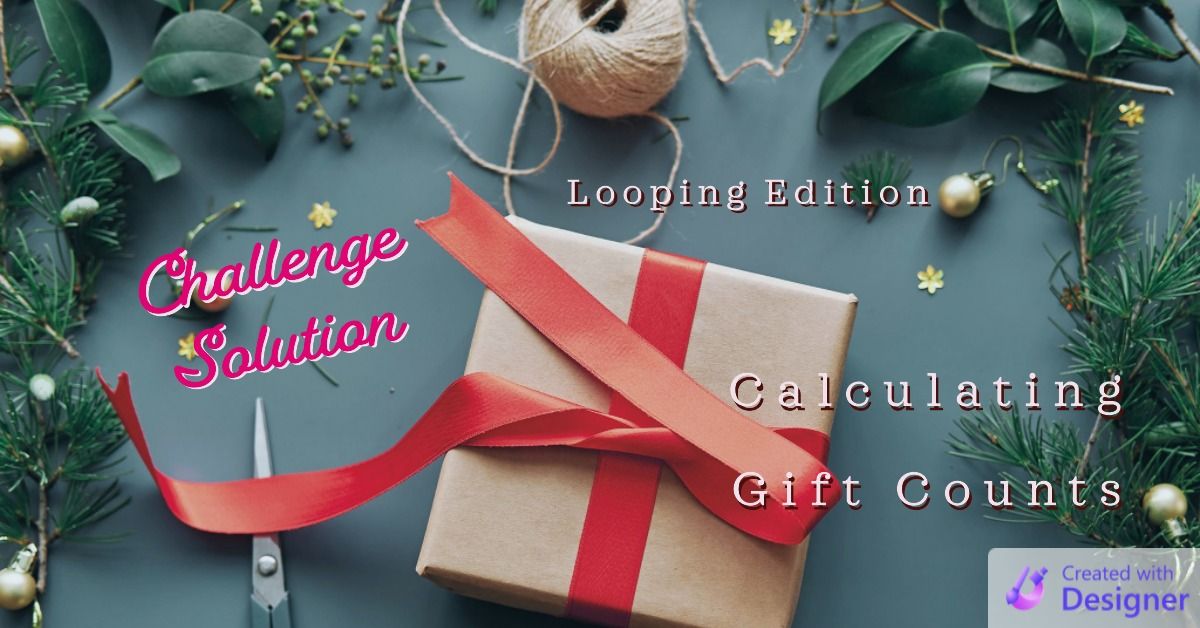
In yesterday's article, I posted a reader challenge:
How many total gifts does one's true love deliver by the nth day of Christmas?
SPOILER ALERT: If you would like to try figuring it out on your own, follow the link above to read the original article before scrolling down to see one of my solutions.
The goal is to write a function that takes as input the first column (Day of Christmas) and returns as output the last column (Cumulative gifts):
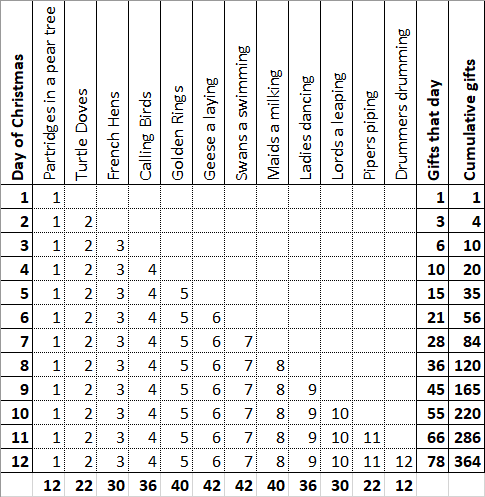
Using a Loop to Calculate the Total
This solution is based on the following pattern that emerges when one studies the above matrix:
1: (1 × 1)
2: (1 × 2) + (2 × 1)
3: (1 × 3) + (2 × 2) + (3 × 1)
4: (1 × 4) + (2 × 3) + (3 × 2) + (4 × 1)
5: (1 × 5) + (2 × 4) + (3 × 3) + (4 × 2) + (5 × 1)
6: (1 × 6) + (2 × 5) + (3 × 4) + (4 × 3) + (5 × 2) + (6 × 1)
If you notice, the first number in each multiplication couplet increments from 1
to n
and the second number in each couplet decrements from n
to 1
.
Based on the above pattern, the solution below goes through a loop n
times and accumulates the total number of gifts given up to and including on day n
.
'--== Looping Version ==--
Function GiftsByXmasDay(DayOfXmas As Long) As Long
'Initialize x and y
Dim x As Long: x = DayOfXmas
Dim y As Long: y = 1
Dim i As Integer
For i = 1 To DayOfXmas
GiftsByXmasDay = GiftsByXmasDay + x * y
x = x - 1 'Decrement x
y = y + 1 'Increment y
Next i
End Function
Referenced articles
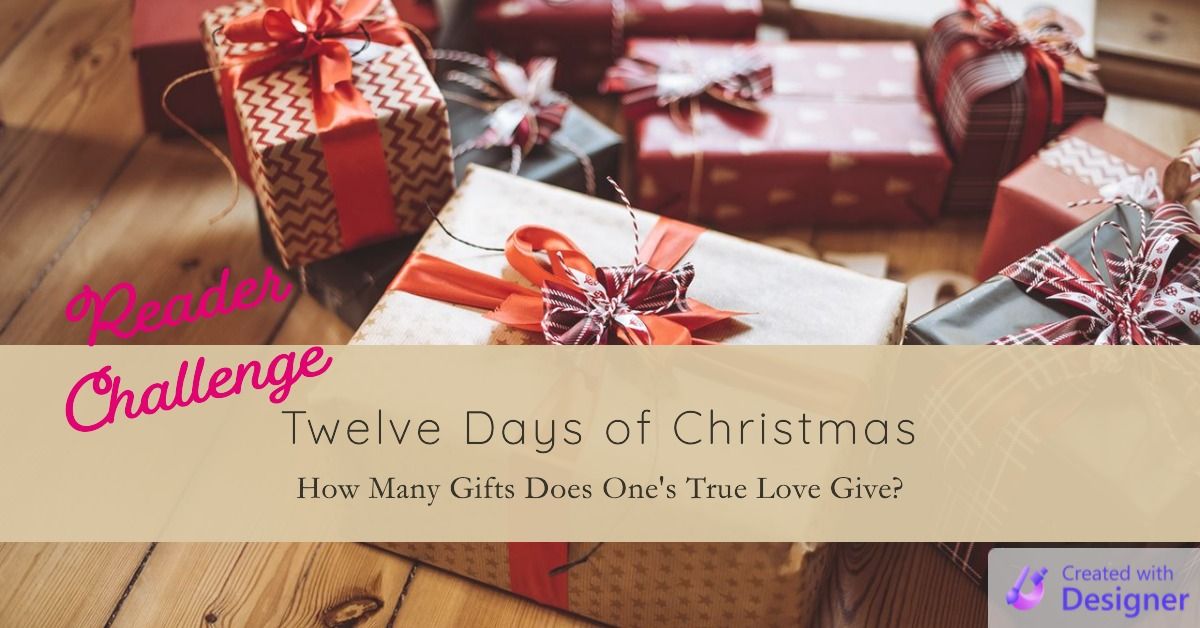
Cover image created with Microsoft Designer