Compile Errors
Compile errors are easy to keep out of deployed code (just remember to Debug > Compile). Which is good because they hard crash Access in Runtime mode.
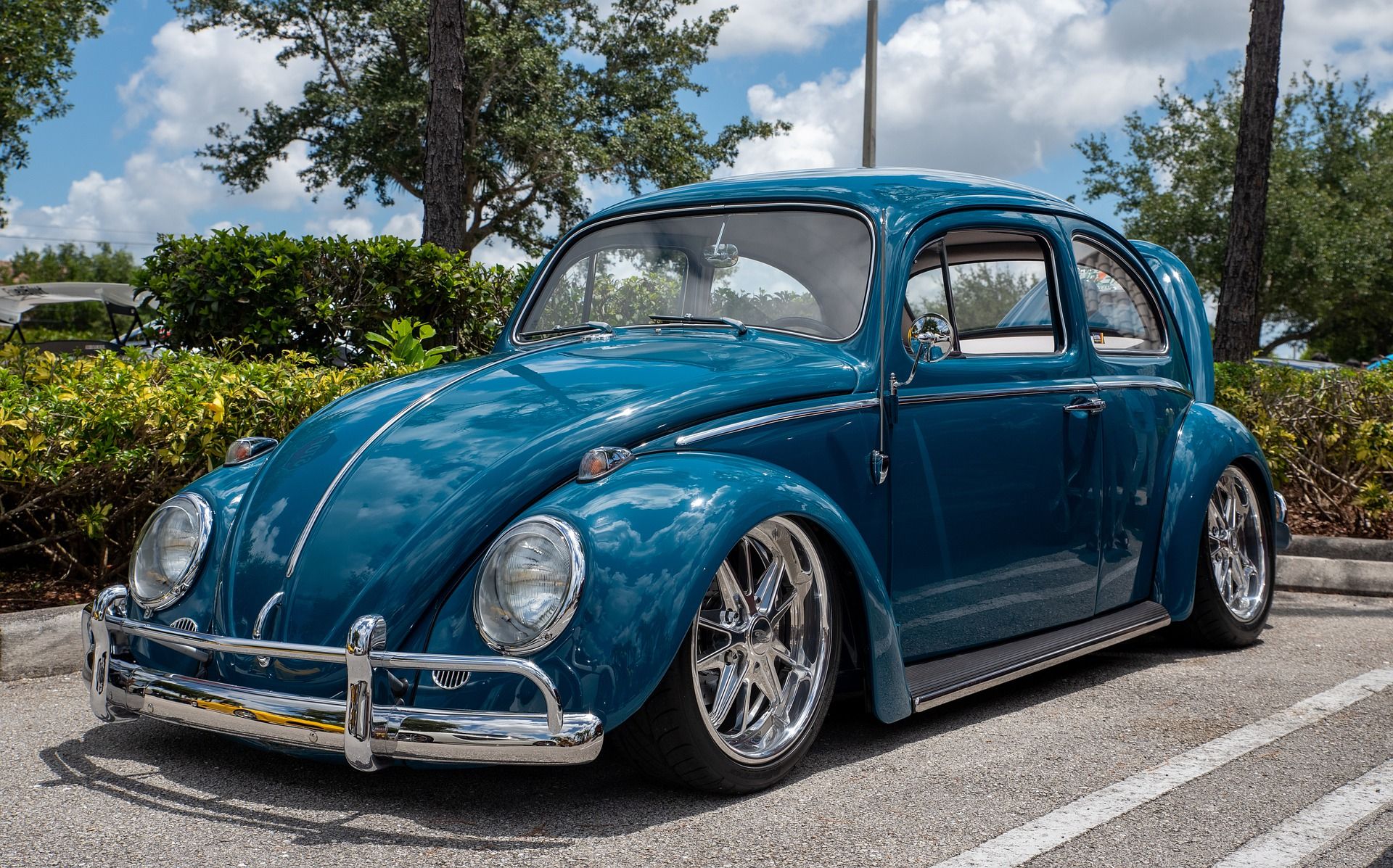
This is part 2 of a 7-part series comparing different kinds of software bugs.
What is a Compile Error?
Visual Basic for Applications (VBA) is a high-level programming language.
Processors have a limited set of instructions that they can execute. The process of translating high-level (human-friendly) programming language code into low-level (CPU-friendly) machine code is known as compilation.
NOTE: When you "compile" VBA, you're not actually compiling it all the way down to machine code. Rather, you are converting it into an intermediate layer known as byte-code or "P-Code". But that's a story for another day.
Translating high-level code to low-level instructions is a computationally expensive process. The main reason for compiling code is to improve runtime performance. A side benefit is that compiled code is harder to plagiarize, though I personally think this is overrated (especially for custom software).
Causes of Compile Errors
As part of the compilation process, VBA will check to make sure that the code you wrote will run when translated into machine code.
For example, if you write a subroutine that takes a String
as an argument passed by reference, but then try to call it with a variable of the Date
data type, VBA will complain when you attempt to compile the code.
That's because when the code executes, the MyDay
variable and the Bar
variable will both be pointing to the same address in memory. A memory location cannot simultaneously be two different data types, so the VBA compiler raises an error.
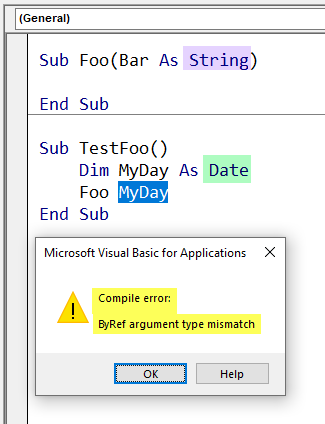
(ByVal Bar As String)
or call Foo with a literal date (#8/6/2021#
) or wrap the MyDay variable in parentheses Foo (MyDay)
, then the compile error goes away. (Note: I'm not suggesting those are fixes! Rather, I'm pointing out another quirk of the ByRef vs. ByVal dynamic).Note that in the screenshot above, none of the text is highlighted in red. That's because the above code is an example of a compile error, but it is not a syntax error.
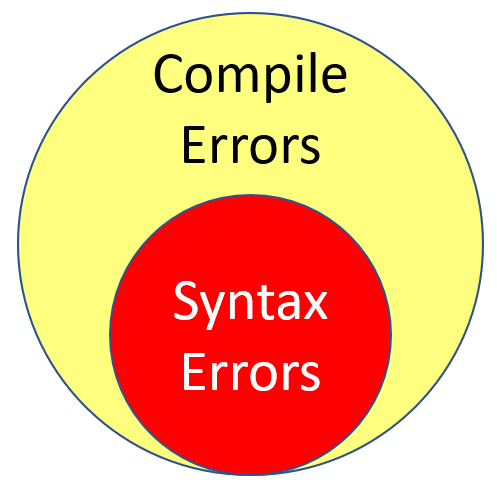
Consequences of Compile Errors
Non-syntax compile errors are not immediately made obvious while you are writing your code.
To force a check for compile errors, you can go to Debug > Compile ([Alt] + [D], [L]). This is a good habit to get into while writing VBA. The compile process runs extremely fast in VBA (under one second) even for very large projects. That's mainly because only changed modules get compiled.
There is one major caveat. If you forget to explicitly compile your code, then you run the risk of publishing a front-end file with an embedded compile error. If that happens, then Access will hard crash in Runtime mode when it hits that error even if you are using vbWatchdog.
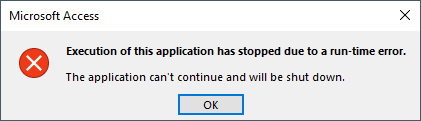
Here's some sample code and a short video that illustrates the difference in how vbWatchdog automatically handles a runtime error versus what happens when your code encounters a compile error.
'--== code-behind of the auto-opening form ==--
Option Compare Database
Option Explicit
Private Sub Form_Load()
ErrEx.Enable "Dummy" 'Step 1. Enable vbWatchdog
MsgBox "test" 'Step 2. Show message box
Debug.Print 1 / 0 'Step 3. Runtime error
TestFoo 'Step 4. Compile error --> hard crash
End Sub
Sub Foo(Bar As String)
End Sub
Sub TestFoo()
Dim MyDay As Date
Foo MyDay
End Sub
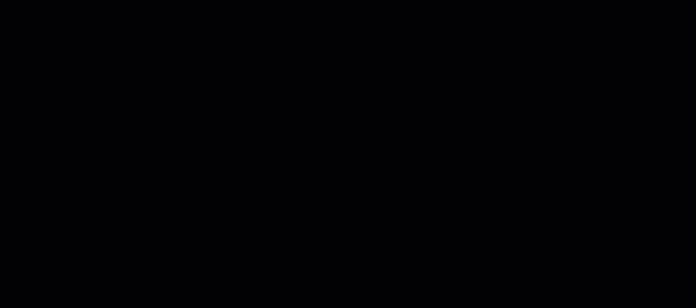
External references


Referenced articles
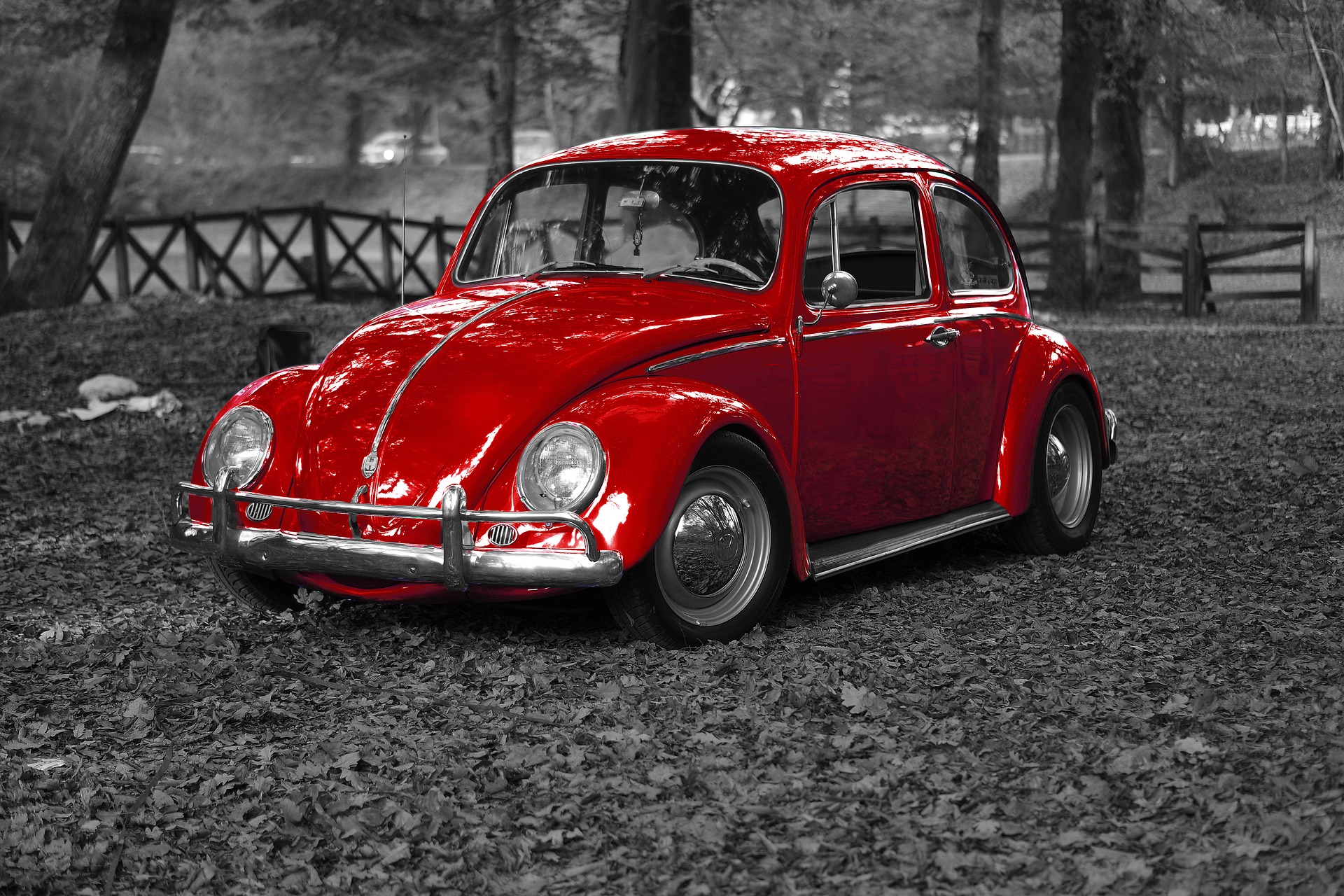
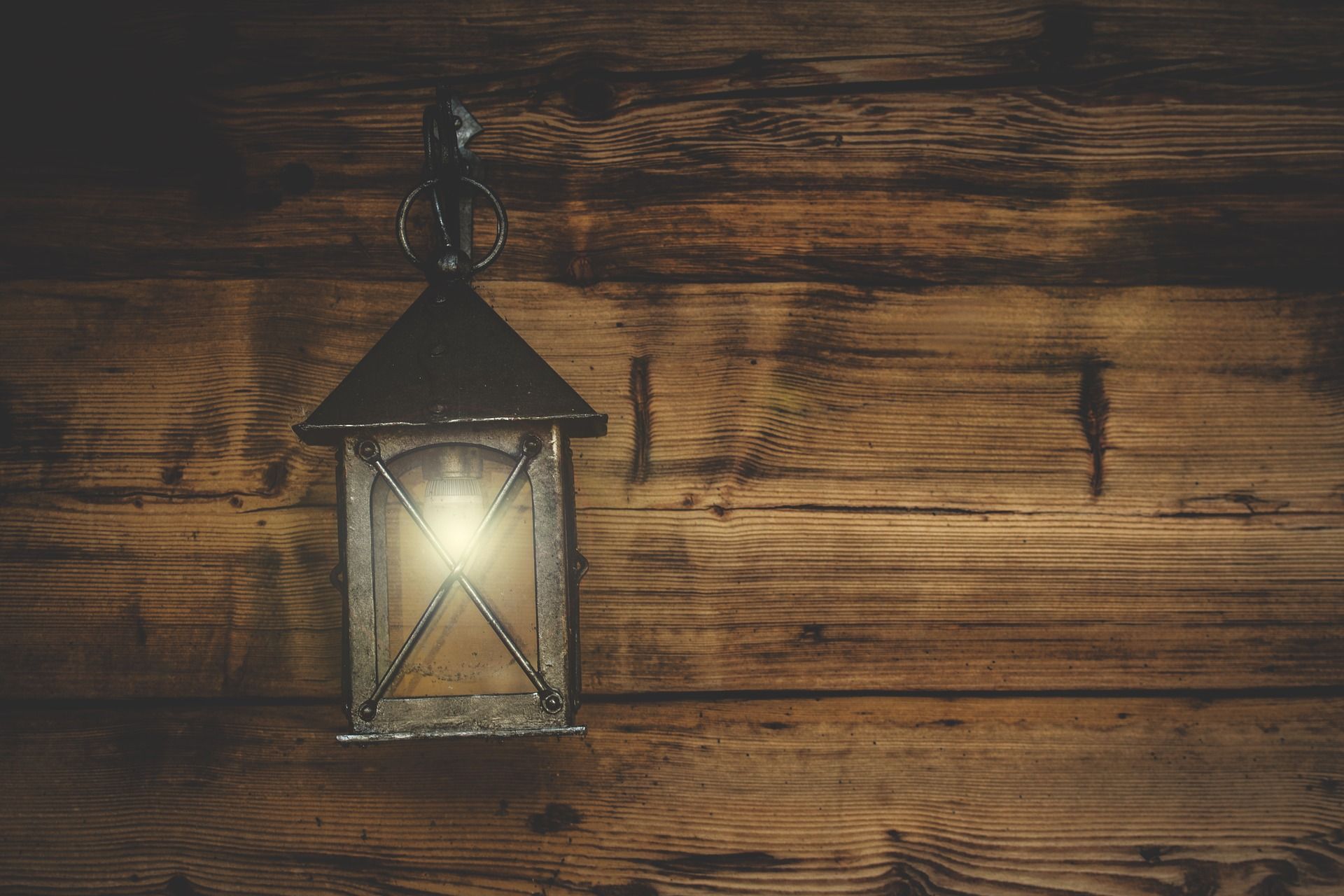
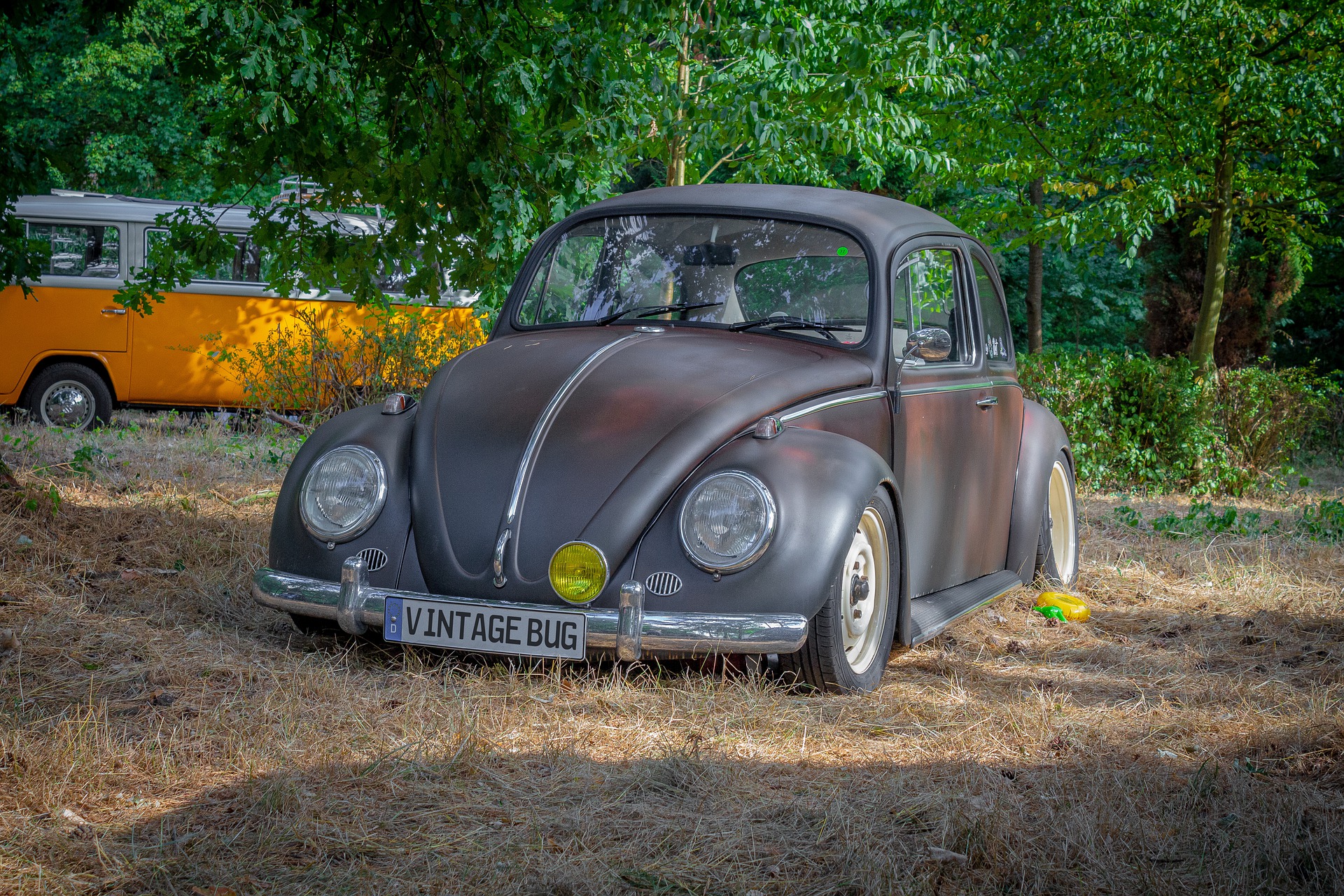
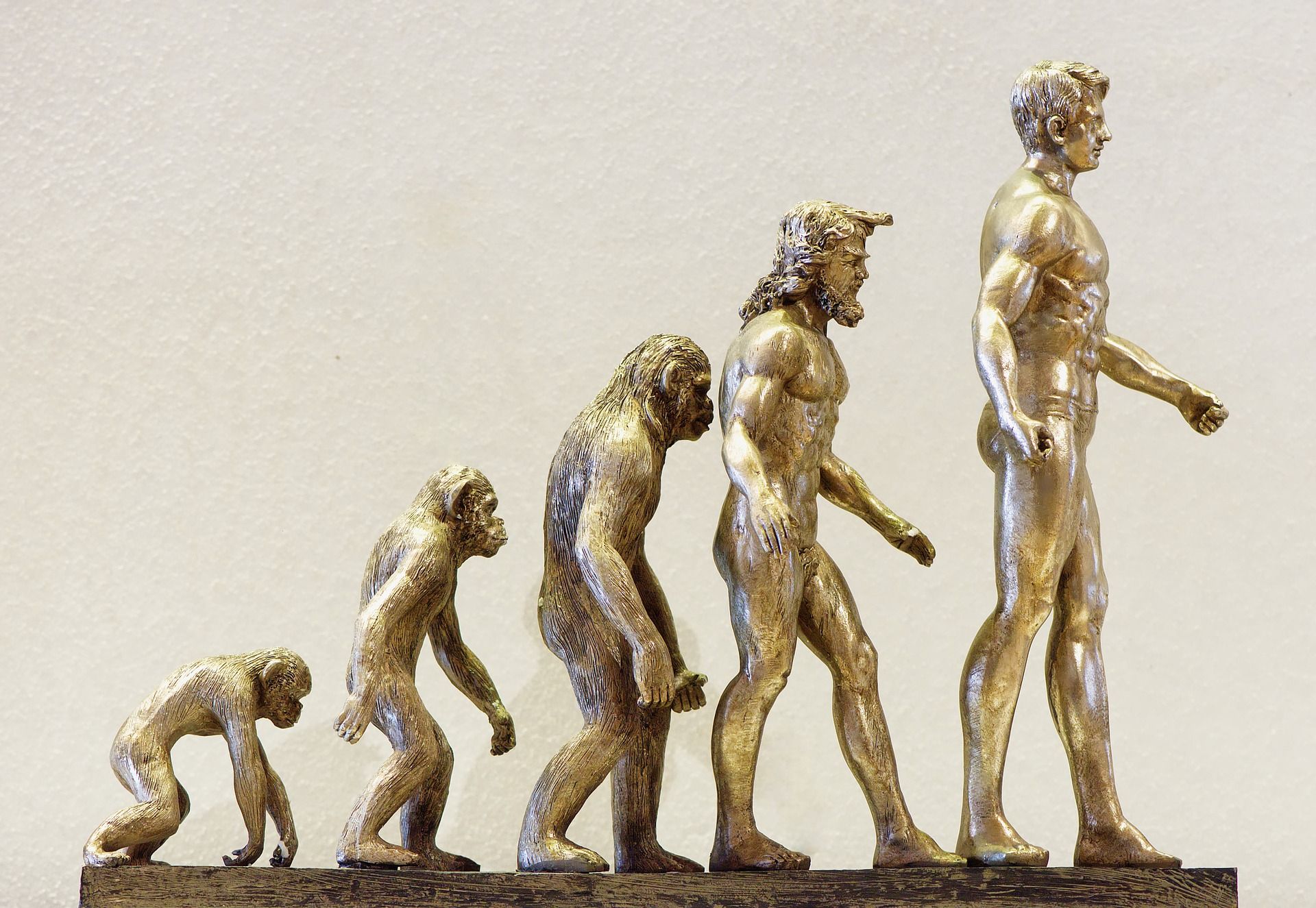
Image by Ernie A. Stephens from Pixabay