3 Ways to Get and Set the TitleBar Text for an MS Access Application
Here are three ways to set the Application Title for an MS Access application. My preferred method allows getting and setting with a single line of code.
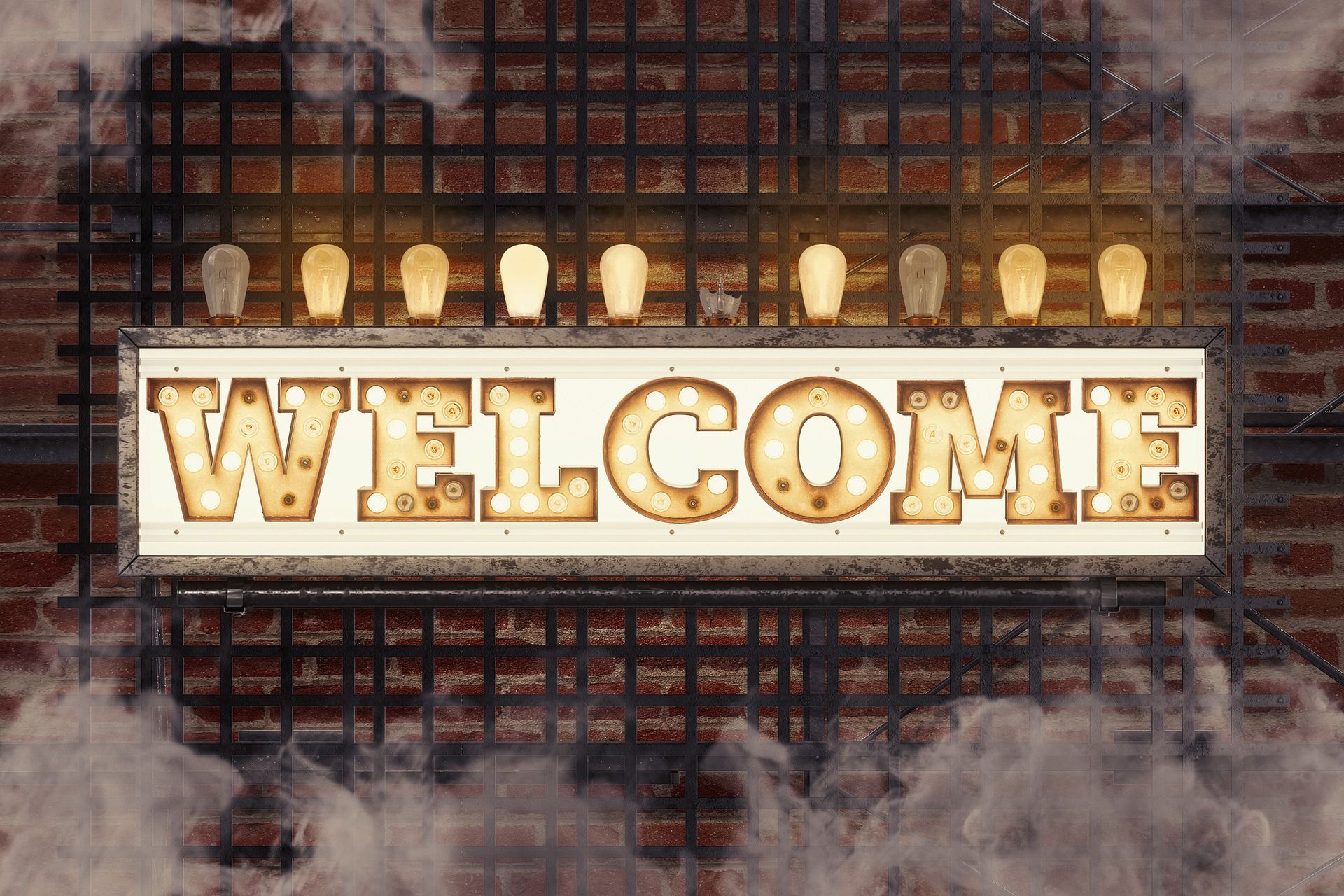
There are three ways to get and set custom title bar text for your Microsoft Access application:
- Via the Options menu
- Using VBA to directly set the "AppTitle" database property
- Using my clsApp class module's
.TitleBar
property
Using the Options Menu
File > Options > Current Database > Application Title
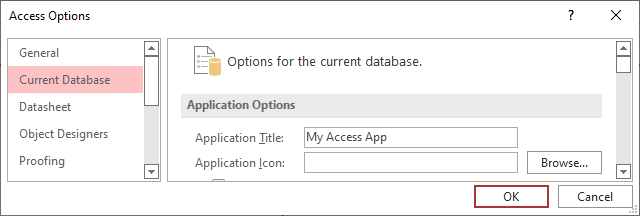
Using VBA to Set the "AppTitle" Property
To get the value of the custom Application Title in code, you can reference the "AppTitle" property of the current database.
?CurrentDB.Properties("AppTitle")
My Access App
To set a custom Application Title in code, you can assign a new value to the "AppTitle" property directly. You will also need to call the RefreshTitleBar
method of the Access.Application object to force the change to take effect:
CurrentDB.Properties("AppTitle") = "My Improved Access App"
RefreshTitleBar
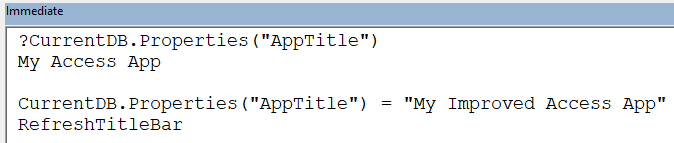
Be careful, though! If you've never set the Application Title in the Options menu for the current database, then the "AppTitle" property will not exist. Trying to access it will return a run-time error:
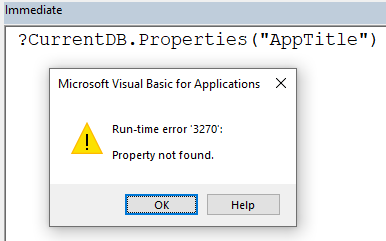
You'll get the same error if you try to write to the property before it's been set in the Options menu:
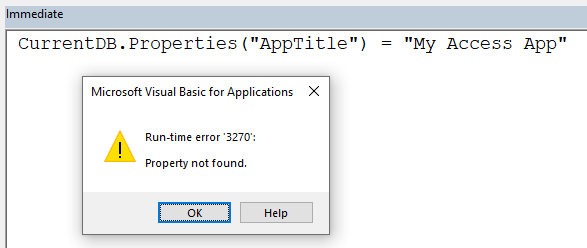
You can still set the property in code, but you will have to call the CreateProperty
method of the current database to create the AppTitle
property and then Append
it to the database's Properties
collection:

Unfortunately, you can't just use the CreateProperty
/ Append
approach blindly, as it will raise a different error if the property already exists:
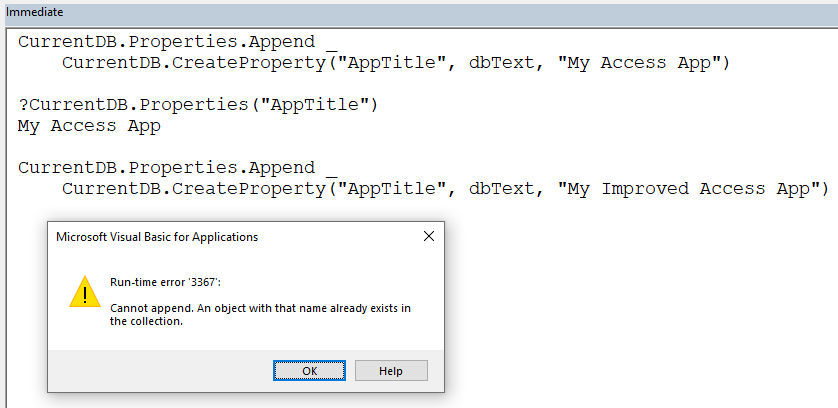
None of these are particularly difficult challenges to overcome, but why reinvent the wheel?
Using clsApp's TitleBar Property
Every application I write contains a singleton class named clsApp.
With this approach, getting (or setting) the title bar is as easy as updating a String property of the App
object instance:
App.TitleBar = "My Access App"
Debug.Print App.TitleBar
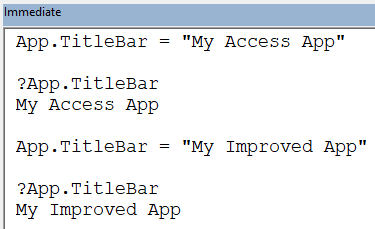
You don't have to worry about whether the property already exists, or to use the correct property name, or to risk a typo that won't get caught at compile time, or remember to refresh the title bar, etc.
The Code
For details about using clsApp, refer to the main clsApp article, which includes full source code for the clsApp class module (including the TitleBar
property shown below).
Public Property Get TitleBar() As String
TitleBar = Me.Prop("AppTitle", "Microsoft Access")
End Property
Public Property Let TitleBar(ByVal sTitleBar As String)
Me.Prop("AppTitle") = sTitleBar
Application.RefreshTitleBar
End Property
Referenced articles
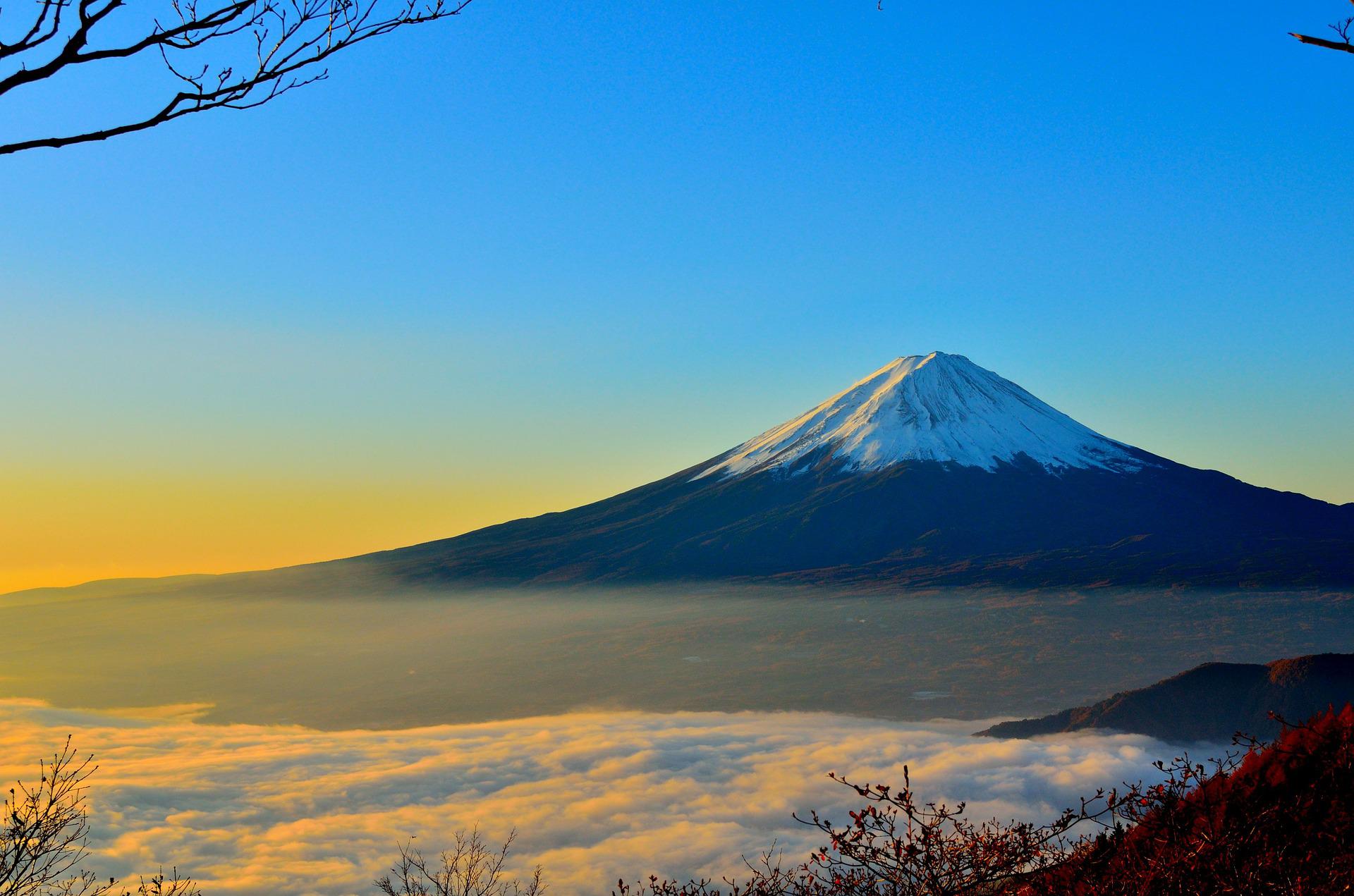
External references
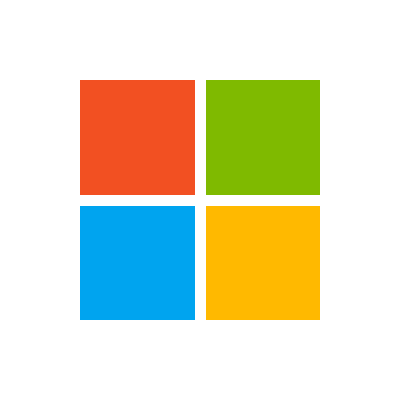